python四叉树图像分割
时间: 2023-09-26 13:14:57 浏览: 223
对于Python中的四叉树图像分割,您可以使用一些第三方库来实现。其中一个流行的库是scikit-image。以下是一个简单的示例代码,演示了如何使用四叉树分割图像:
```python
import numpy as np
from skimage import io, color, util
class QuadTreeNode:
def __init__(self, image, threshold):
self.image = image
self.threshold = threshold
self.children = []
def split(self):
height, width = self.image.shape
if height <= 1 or width <= 1:
return
if np.std(self.image) > self.threshold:
# Split the image into four quadrants
h_mid, w_mid = height // 2, width // 2
quadrants = [
self.image[:h_mid, :w_mid],
self.image[h_mid:, :w_mid],
self.image[:h_mid, w_mid:],
self.image[h_mid:, w_mid:]
]
for quadrant in quadrants:
node = QuadTreeNode(quadrant, self.threshold)
node.split()
self.children.append(node)
def get_regions(self):
if not self.children:
return [self.image]
regions = []
for child in self.children:
regions.extend(child.get_regions())
return regions
def image_segmentation(image_path, threshold):
# Load the image
image = io.imread(image_path)
# Convert the image to grayscale
grayscale_image = color.rgb2gray(image)
# Normalize the image to values between 0 and 1
normalized_image = util.img_as_float(grayscale_image)
# Construct the quadtree and split it recursively
root_node = QuadTreeNode(normalized_image, threshold)
root_node.split()
# Get the regions from the quadtree nodes
regions = root_node.get_regions()
# Convert the regions back to the original image scale
scaled_regions = [util.img_as_ubyte(region) for region in regions]
return scaled_regions
# Example usage
image_path = 'path/to/your/image.jpg'
threshold = 0.1
regions = image_segmentation(image_path, threshold)
# Save the regions as individual images
for i, region in enumerate(regions):
io.imsave(f'path/to/save/region_{i}.jpg', region)
```
在上述代码中,我们首先加载图像,并将其转换为灰度图像。然后,我们将图像归一化为0到1之间的值。接下来,我们构建了一个QuadTreeNode类来表示四叉树的节点,并实现了split()方法来递归地分割图像。最后,我们使用get_regions()方法获取分割后的图像区域,并将其保存为单独的图像文件。
请注意,这只是一个简单的示例,您可以根据您的需求进行修改和扩展。希望对您有帮助!
阅读全文
相关推荐
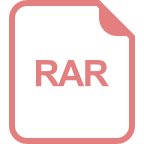
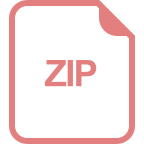
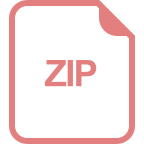
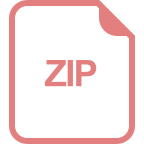
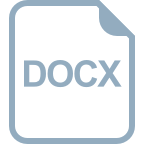
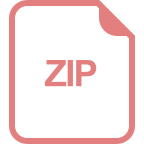
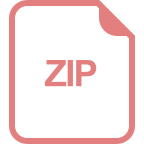
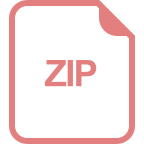
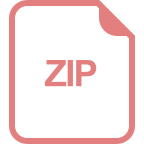
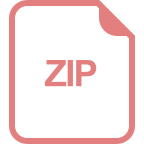
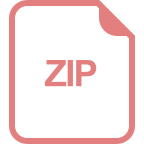
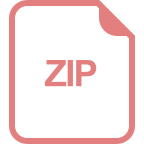