一个文本文件记录了一个矩形和一个不规则图形的坐标,文本记录内容如下 1 1,2,3 3,4 4,5 2 2,3 4,3 4,4 文本内容的含义是分别记录了两个图形的坐标 现在C++中编写程序 对这两个图形的坐标信息进行读取并绘制,定义变量a 代表第几个图形,要求利用for 循环 当a=1时循环读取坐标并绘制,当a=2时循环读取坐标进行绘制,最后利用easyx的可视化功能将绘制好的函数显示出来
时间: 2023-09-30 17:04:08 浏览: 75
以下是代码实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <graphics.h>
using namespace std;
// 定义函数绘制矩形
void drawRectangle(vector<int> coordinates) {
// 获取矩形的左上角和右下角坐标
int x1 = coordinates[0], y1 = coordinates[1], x2 = coordinates[2], y2 = coordinates[3];
// 绘制矩形
rectangle(x1, y1, x2, y2);
}
// 定义函数绘制不规则图形
void drawIrregularShape(vector<vector<int>> coordinates) {
// 定义多边形顶点数组
int poly[20];
// 获取不规则图形的顶点坐标
for (int i = 0; i < coordinates.size(); i++) {
poly[i * 2] = coordinates[i][0];
poly[i * 2 + 1] = coordinates[i][1];
}
// 绘制不规则图形
fillpoly(coordinates.size(), poly);
}
int main() {
// 初始化easyx图形界面
initgraph(800, 600);
// 读取文本文件
ifstream inputFile("coordinates.txt");
string line;
// 定义坐标数组和坐标矩阵
vector<int> coordinates(4);
vector<vector<int>> matrix;
// 定义计数器和图形标志
int count = 0, a = 0;
// 循环读取文本文件中的坐标信息
while (getline(inputFile, line)) {
// 将字符串转换为整数
int num;
vector<int> temp;
for (int i = 0; i < line.size(); i++) {
if (line[i] == ' ' || line[i] == ',') {
temp.push_back(num);
num = 0;
} else {
num = num * 10 + (line[i] - '0');
}
}
temp.push_back(num);
// 如果是第一行,则代表读取的是矩形坐标
if (count == 0) {
coordinates = temp;
} else {
// 否则代表读取的是不规则图形坐标
matrix.push_back(temp);
}
// 如果已经读取了两行,则代表一个图形坐标读取完成,开始绘制
if (count == 1) {
// 判断当前绘制的是哪个图形
if (a == 1) {
drawRectangle(coordinates);
} else if (a == 2) {
drawIrregularShape(matrix);
}
// 重置计数器和坐标数组和坐标矩阵
count = 0;
coordinates.clear();
matrix.clear();
} else {
count++;
}
// 如果当前是第二个图形的坐标读取,则重置图形标志
if (a == 2) {
a = 0;
}
}
// 关闭easyx图形界面
closegraph();
return 0;
}
```
在上述代码中,我们首先初始化了easyx图形界面,并且读取了文本文件中的坐标信息。然后我们定义了两个函数,分别用于绘制矩形和不规则图形。在主函数中,我们使用循环读取坐标信息,并根据a的值选择绘制矩形还是不规则图形。最后我们关闭easyx图形界面,程序执行完毕。
阅读全文
相关推荐
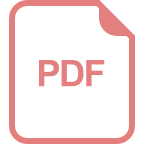
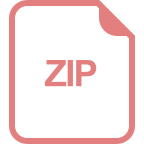
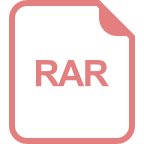
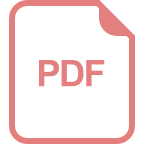
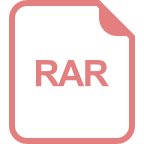
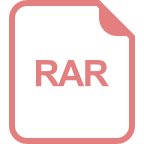
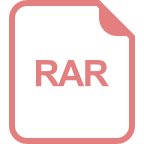
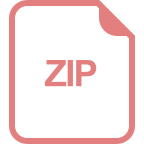
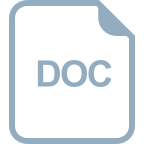
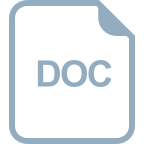
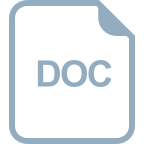
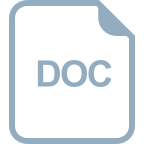
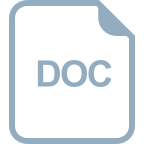
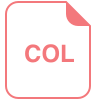
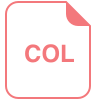
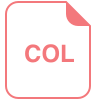
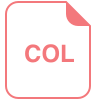
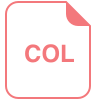
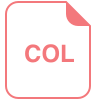
