有一个文本文件,每一行记录一对坐标点,第一行到第四行代表的是矩形的坐标点,第五行到第九行代表的是不规则图形的坐标点,现在C++ 中编写程序读取坐标信息,对于一到四行采用矩形算法函数进行绘制,对于五到九行采用不规则算法函数进行绘制 最后利用easyx的可视化功能将绘制好的函数显示出来 要求程序能够在C++ 中成功运行)(解决法案5
时间: 2023-12-10 15:38:01 浏览: 291
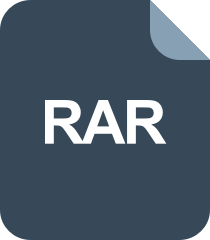
area MATLAB.rar_matlab 面积_房产面积计算_点位坐标算面积_面积计算
以下是一个示例程序,实现了读取坐标信息、绘制矩形和不规则图形,并使用EasyX库进行可视化显示:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <graphics.h>
using namespace std;
// 矩形绘制函数
void drawRectangle(int x1, int y1, int x2, int y2)
{
rectangle(x1, y1, x2, y2);
}
// 不规则图形绘制函数
void drawIrregular(vector<int>& coords)
{
int n = coords.size() / 2;
int* points = new int[n * 2];
for (int i = 0; i < n; i++) {
points[i * 2] = coords[i * 2];
points[i * 2 + 1] = coords[i * 2 + 1];
}
drawpoly(n, points);
delete[] points;
}
int main()
{
// 读取文件
ifstream inFile("coords.txt");
vector<int> coords;
int x, y;
while (inFile >> x >> y) {
coords.push_back(x);
coords.push_back(y);
}
inFile.close();
// 初始化图形界面
initgraph(800, 600);
// 绘制矩形
drawRectangle(coords[0], coords[1], coords[2], coords[3]);
// 绘制不规则图形
vector<int> irregularCoords(coords.begin() + 4, coords.end());
drawIrregular(irregularCoords);
// 显示图形界面
getch();
closegraph();
return 0;
}
```
其中,`coords.txt`文件的内容应该如下所示:
```
100 100
200 100
200 200
100 200
300 300
400 200
500 300
450 400
350 400
```
这个示例程序中,我们首先读取文件中的所有坐标点,并将它们存储在一个`vector<int>`中。然后,我们使用EasyX库提供的`rectangle`和`drawpoly`函数来分别绘制矩形和不规则图形。
需要注意的是,我们在绘制不规则图形时,需要将坐标点从`vector`中提取出来,并将它们转换为一个数组,以便传递给`drawpoly`函数。
最后,我们使用EasyX库提供的`getch`和`closegraph`函数来显示图形界面,并等待用户按下任意键关闭图形界面。
阅读全文
相关推荐
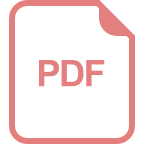
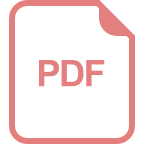
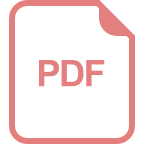
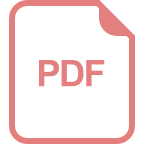
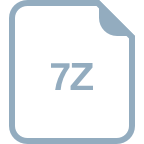
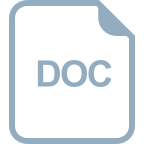
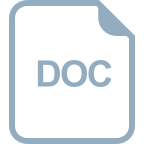
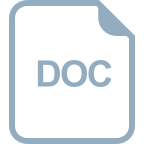
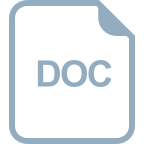
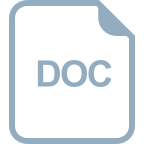
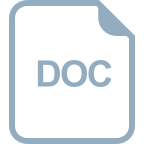
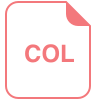
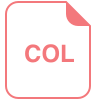
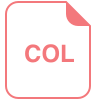
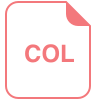
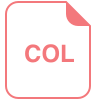
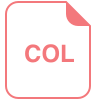
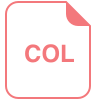