[Error] cannot convert 'BiTree' {aka 'BiTNode*'} to 'BiTNode**' in assignment
时间: 2023-12-18 14:03:33 浏览: 332
这个错误通常是因为您在对指针进行赋值时,类型不匹配导致的。具体来说,在C/C++中,指针是一种特殊的变量类型,它存储了另一个变量的地址。指针变量本身存储在内存中,而它指向的变量也存储在内存中,但是它们在内存中的位置是不同的。
在您的代码中,出现了将一个指向 BiTNode 结构体的指针赋值给一个指向指针的变量的情况。这通常会导致类型不匹配的错误。请检查您的代码,确认您是否正确地声明和使用了指针变量,并且在进行赋值操作时,将指向 BiTNode 结构体的指针赋值给指向指针的变量。
相关问题
typedef struct BiTNodefElemType data ; struct BiTNode * lchild , * rchild ; BiTNode * BiTree ;
这段代码是一个二叉树的定义和声明,其中包含了一个结构体 `BiTNode` 和一个指向该结构体的指针 `BiTree`。结构体 `BiTNode` 包含了一个成员变量 `data`,用来存储节点的数据,以及两个指向左子树和右子树的指针 `lchild` 和 `rchild`。
`typedef struct BiTNodefElemType data;` 这行代码是对 `data` 的数据类型进行定义,但是你没有给出 `data` 的具体类型。如果你想要使用这段代码,需要在 `typedef` 之前定义 `data` 的数据类型。
然后,`struct BiTNode * lchild, * rchild;` 这行代码声明了两个指向 `struct BiTNode` 类型的指针,分别表示左子树和右子树。
最后,`BiTNode * BiTree;` 这行代码声明了一个指向 `struct BiTNode` 类型的指针 `BiTree`,用来表示整个二叉树的根节点。
需要注意的是,你在代码中使用了 `BiTNode *` 来声明指针变量,但是在结构体定义中使用了 `struct BiTNode *`。这可能是一个笔误,请确保在代码的其他部分中使用一致的命名。
已知在以二叉链表存储的二叉树t中,p、q为二叉树中两个不相同结点,编写算法求包含结点 p和q的最小子树根结点。初始化堆栈InitStack(S) typedef struct BiTNode TElemType data; Struct BiTNode *Ichild, *rchild; ] BiTNode , *BiTree; 算法编制,BiTNode*suanfa(BiTree bt, BiTNode *p, BiTNode *q)
好的,以下是代码实现:
```
typedef struct {
BiTree bt;
BiTNode *node;
int tag;
} SNode;
void findPath(BiTree bt, BiTNode *node, Stack &S) {
if (bt == NULL) return;
if (bt == node) { // 找到目标结点,将其入栈并返回
Push(S, SNode{bt, NULL, 1});
return;
}
findPath(bt->lchild, node, S); // 在左子树中查找
findPath(bt->rchild, node, S); // 在右子树中查找
if (!StackEmpty(S)) { // 如果找到了目标结点,则将当前结点入栈
SNode top;
GetTop(S, top);
if (top.tag == 1) {
Push(S, SNode{bt, NULL, 0});
}
}
}
BiTNode* suanfa(BiTree bt, BiTNode *p, BiTNode *q) {
Stack S;
InitStack(S);
findPath(bt, p, S); // 找到包含p的路径
findPath(bt, q, S); // 找到包含q的路径
BiTNode *last = NULL; // 记录上一个访问的结点
while (!StackEmpty(S)) {
SNode top;
Pop(S, top);
if (top.tag == 1) { // 第一次访问该结点,将其右子树入栈
top.tag = 0;
Push(S, top);
if (last != NULL) {
return last; // 如果已经访问过一个结点了,且当前结点为其右子树,那么上一个结点就是公共祖先
}
} else { // 第二次访问该结点,将其左子树入栈
if (top.node->lchild == last) {
return top.node; // 如果已经访问过一个结点了,且当前结点为其左子树,那么该结点就是公共祖先
}
}
last = top.node; // 记录上一个访问的结点
}
return NULL; // 没有找到公共祖先
}
```
解释如下:
输入参数:
- bt: 二叉树的根结点指针
- p: 目标结点p的指针
- q: 目标结点q的指针
返回值:
- 返回包含结点p和q的最小子树根结点的指针,如果不存在这样的根结点,返回NULL。
实现方式:
- 定义一个辅助结构体SNode,用于记录结点、指针和访问标记。
- 定义一个辅助函数findPath,用于在二叉树中查找包含目标结点的路径,并将路径上的结点入栈。
- 在主函数suanfa中,首先初始化一个堆栈S。
- 分别调用findPath函数查找包含p和q的路径,并将路径上的结点入栈。
- 定义一个指针变量last,用于记录上一个访问的结点。
- 在堆栈S中遍历元素,如果当前元素的tag为1,表示第一次访问该结点,那么将其右子树入栈,并将访问标记标记为0;如果当前元素的tag为0,表示第二次访问该结点,那么将其左子树入栈。
- 如果已经访问过一个结点了,且当前结点为其右子树,那么上一个结点就是公共祖先;如果已经访问过一个结点了,且当前结点为其左子树,那么该结点就是公共祖先。
- 最后,如果没有找到公共祖先,返回NULL。
注意:上述代码中使用了一个辅助栈S来实现查找路径和回溯的过程,这是因为二叉树的路径并不是线性的,不能直接记录路径,需要通过栈来保存路径上的结点。同时,在回溯的过程中,需要判断当前结点是在其父结点的左子树还是右子树中,这也需要通过栈来实现。
相关推荐
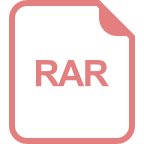












