python img.draw_string
时间: 2024-04-09 19:27:52 浏览: 179
Python的img.draw_string()函数是用于在图像上绘制字符串的方法。它通常与图像处理库(如PIL或OpenCV)一起使用。
例如,在PIL库中,可以使用ImageDraw模块的text()方法来绘制字符串。下面是一个示例:
```python
from PIL import Image, ImageDraw, ImageFont
# 打开图像
image = Image.open("image.jpg")
# 创建可绘制对象
draw = ImageDraw.Draw(image)
# 设置字体
font = ImageFont.truetype("arial.ttf", size=20)
# 绘制字符串
text = "Hello, World!"
position = (50, 50)
color = (255, 0, 0) # 字体颜色为红色
draw.text(position, text, font=font, fill=color)
# 保存修改后的图像
image.save("modified_image.jpg")
```
在上面的示例中,我们首先打开了一个图像,然后创建了一个可绘制对象。接下来,我们选择了一个字体并设置了绘制位置和颜色。最后,我们使用draw.text()方法将字符串绘制到图像上,并将修改后的图像保存到新文件中。
请注意,上面的示例使用了PIL库,如果您使用的是其他图像处理库,可能会有一些差异。
相关问题
img.draw_string用法
`img.draw_string()`是MicroPython中的一个函数,用于在图像上绘制文本。它的用法如下:
```python
img.draw_string(x, y, text, color=None, scale=1, mono_space=False, fixedwidth=False)
```
参数说明:
- `x`:文本左上角的x坐标。
- `y`:文本左上角的y坐标。
- `text`:要绘制的文本内容。
- `color`:文本颜色,默认为图片的颜色。
- `scale`:文本缩放比例,默认为1。
- `mono_space`:是否开启等宽模式,默认为False。
- `fixedwidth`:是否使用固定宽度,默认为False。
示例:
```python
import sensor, image, lcd
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
lcd.init()
img = sensor.snapshot()
# 在图像上绘制文本
img.draw_string(10, 10, "Hello World!", color=(255, 0, 0), scale=2)
lcd.display(img)
```
运行结果:

img.draw_string的用法
img.draw_string() 是 MicroPython 的一个图形库中的函数,用于在图像上绘制字符串。
函数定义:
```python
img.draw_string(x, y, string, color[, scale[, mono_space]])
```
参数说明:
- x:字符串在图像中的横坐标起始位置;
- y:字符串在图像中的纵坐标起始位置;
- string:要绘制的字符串;
- color:字符串的颜色;
- scale(可选):字符串的缩放比例,默认为 1;
- mono_space(可选):是否使用等宽字体,默认为 False。
示例用法:
```python
import sensor, image
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(10)
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
img = sensor.snapshot()
img.draw_string(10, 10, "Hello, world!", color=(255, 0, 0), scale=2, mono_space=True)
img.draw_string(10, 50, "MicroPython", color=(0, 255, 0), scale=1)
img.draw_string(10, 100, "OpenMV", color=(0, 0, 255), scale=3)
while True:
pass
```
上述代码中,我们首先初始化了摄像头,然后拍摄一张图片,接着在图片上分别绘制了三个字符串,最后将图片显示在屏幕上。运行程序后,你应该能够看到如下效果:

阅读全文
相关推荐
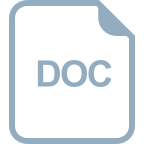
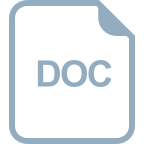
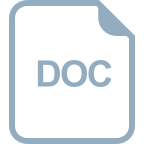






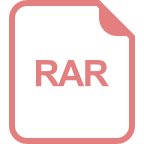
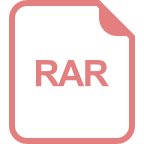
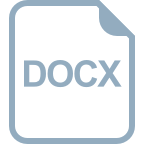
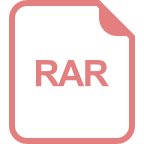



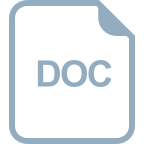