STM32F103和STM32F407的DMA配置有什么区别,请写一个示例程序
时间: 2023-12-23 08:02:09 浏览: 31
STM32F103和STM32F407的DMA配置有以下区别:
1. STM32F407支持更高速的DMA传输,最高可达168MHz;
2. STM32F407支持更多的DMA通道,可达16个通道,而STM32F103只有7个通道;
3. STM32F407支持多缓存区模式,可以实现双缓存或循环缓存的DMA传输;
4. STM32F407支持更多的外设,如USB、SDIO等,这些外设都可以使用DMA传输。
以下是一个基于STM32CubeIDE的示例程序,用于将ADC采集的数据通过DMA传输到SRAM中:
```c
#include "stm32f1xx_hal.h"
#include "stm32f1xx_hal_adc.h"
#include "stm32f1xx_hal_dma.h"
#define ADC_BUFFER_SIZE 100
/* ADC handle declaration */
ADC_HandleTypeDef hadc1;
/* DMA handle declaration */
DMA_HandleTypeDef hdma_adc1;
/* Buffer used for ADC conversion */
uint16_t adc_buffer[ADC_BUFFER_SIZE];
/* Function prototypes */
static void MX_DMA_Init(void);
static void MX_ADC1_Init(void);
int main(void)
{
/* MCU Configuration--------------------------------------------------------*/
/* Reset of all peripherals, Initializes the Flash interface and the Systick. */
HAL_Init();
/* Configure the system clock */
SystemClock_Config();
/* Initialize all configured peripherals */
MX_DMA_Init();
MX_ADC1_Init();
/* Start ADC conversion */
HAL_ADC_Start_DMA(&hadc1, (uint32_t*)adc_buffer, ADC_BUFFER_SIZE);
while (1)
{
/* Do something with the ADC data */
}
}
/**
* Initializes the DMA controller for ADC channel 1.
*/
static void MX_DMA_Init(void)
{
/* DMA controller clock enable */
__HAL_RCC_DMA1_CLK_ENABLE();
/* DMA interrupt init */
/* DMA1_Channel1_IRQn interrupt configuration */
HAL_NVIC_SetPriority(DMA1_Channel1_IRQn, 0, 0);
HAL_NVIC_EnableIRQ(DMA1_Channel1_IRQn);
/* DMA1 channel1 configuration */
hdma_adc1.Instance = DMA1_Channel1;
hdma_adc1.Init.Direction = DMA_DIR_PERIPH_TO_MEMORY; // STM32F103和STM32F407的DMA配置区别之一,传输方向不同
hdma_adc1.Init.PeriphInc = DMA_PINC_DISABLE;
hdma_adc1.Init.MemInc = DMA_MINC_ENABLE;
hdma_adc1.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD;
hdma_adc1.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD;
hdma_adc1.Init.Mode = DMA_CIRCULAR; // STM32F407支持多缓存区模式,STM32F103不支持
hdma_adc1.Init.Priority = DMA_PRIORITY_HIGH;
if (HAL_DMA_Init(&hdma_adc1) != HAL_OK)
{
Error_Handler();
}
/* DMA interrupt init */
/* DMA1_Channel1_IRQn interrupt configuration */
HAL_NVIC_SetPriority(DMA1_Channel1_IRQn, 0, 0);
HAL_NVIC_EnableIRQ(DMA1_Channel1_IRQn);
}
/**
* Initializes the ADC1 channel 1 with DMA.
*/
static void MX_ADC1_Init(void)
{
ADC_ChannelConfTypeDef sConfig = {0};
/* ADC1 clock enable */
__HAL_RCC_ADC1_CLK_ENABLE();
/* DMA1 clock enable */
__HAL_RCC_DMA1_CLK_ENABLE();
/* ADC1 DMA Init */
/* ADC1 Init */
hadc1.Instance = ADC1;
hadc1.Init.ScanConvMode = ADC_SCAN_ENABLE;
hadc1.Init.ContinuousConvMode = ENABLE;
hadc1.Init.DiscontinuousConvMode = DISABLE;
hadc1.Init.ExternalTrigConv = ADC_SOFTWARE_START;
hadc1.Init.DataAlign = ADC_DATAALIGN_RIGHT;
hadc1.Init.NbrOfConversion = 1;
if (HAL_ADC_Init(&hadc1) != HAL_OK)
{
Error_Handler();
}
/* Configure regular channel */
sConfig.Channel = ADC_CHANNEL_1;
sConfig.Rank = ADC_REGULAR_RANK_1;
sConfig.SamplingTime = ADC_SAMPLETIME_13CYCLES_5;
if (HAL_ADC_ConfigChannel(&hadc1, &sConfig) != HAL_OK)
{
Error_Handler();
}
/* ADC1 DMA Init */
hdma_adc1.Instance = DMA1_Channel1;
hdma_adc1.Init.Direction = DMA_DIR_PERIPH_TO_MEMORY; // STM32F103和STM32F407的DMA配置区别之一,传输方向不同
hdma_adc1.Init.PeriphInc = DMA_PINC_DISABLE;
hdma_adc1.Init.MemInc = DMA_MINC_ENABLE;
hdma_adc1.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD;
hdma_adc1.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD;
hdma_adc1.Init.Mode = DMA_CIRCULAR; // STM32F407支持多缓存区模式,STM32F103不支持
hdma_adc1.Init.Priority = DMA_PRIORITY_HIGH;
if (HAL_DMA_Init(&hdma_adc1) != HAL_OK)
{
Error_Handler();
}
/* Associate the initialized DMA handle to the the ADC handle */
__HAL_LINKDMA(&hadc1, DMA_Handle, hdma_adc1);
/* ADC1 interrupt Init */
HAL_NVIC_SetPriority(ADC1_IRQn, 0, 0);
HAL_NVIC_EnableIRQ(ADC1_IRQn);
}
```
这是一个简单的示例程序,仅供参考。具体的DMA配置需要根据实际应用场景进行调整。
相关推荐
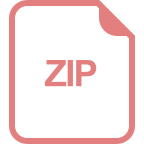
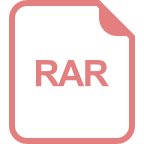














