解释一下这段代码parser = argparse.ArgumentParser() parser.add_argument('--root_path', type=str, default='../data/ACDC', help='Name of Experiment')
时间: 2024-01-27 14:04:22 浏览: 31
这段代码是用来解析命令行参数的。首先,我们创建了一个ArgumentParser对象,它可以帮助我们定义和解析命令行参数。然后,调用add_argument方法来添加我们感兴趣的命令行参数。
在这个例子中,我们添加了一个名为'--root_path'的命令行参数。它的类型是字符串类型(str),默认值是'../data/ACDC',并且还提供了一个帮助文本用于描述这个参数的作用。这个参数用于指定实验的根路径。
当我们运行这个脚本时,可以通过命令行来指定'--root_path'参数的值。例如,我们可以运行以下命令来指定不同的根路径:
```
python script.py --root_path /path/to/experiment
```
在脚本中,我们可以通过访问args.root_path来获取命令行参数的值,以便在后续代码中使用。
相关问题
if __name__ == "__main__": import argparse parser = argparse.ArgumentParser( description=__doc__) # 使用设备类型 parser.add_argument('--device', default='cuda:2', help='device') # 检测目标类别数 parser.add_argument('--num-classes', type=int, default='3', help='number of classes') # 数据集的根目录(VOCdevkit) parser.add_argument('--data-path', default='./', help='dataset root') # 训练好的权重文件 parser.add_argument('--weights-path', default='./save_weights_resNet/resNetFpn-model-0.pth', type=str, help='training weights') # batch size parser.add_argument('--batch_size', default=1, type=int, metavar='N', help='batch size when validation.') args = parser.parse_args() main(args)
这段代码使用 argparse 解析命令行参数,并调用了一个名为 main() 的函数,传入参数 args。
具体解析的参数包括:
- `--device`:用于指定设备类型,默认值为 'cuda:2'。
- `--num-classes`:用于指定检测目标的类别数,默认值为 3。
- `--data-path`:用于指定数据集的根目录,默认值为 './'。
- `--weights-path`:用于指定训练好的权重文件的路径,默认值为 './save_weights_resNet/resNetFpn-model-0.pth'。
- `--batch_size`:用于指定验证时的批处理大小,默认值为 1。
你可以根据需要修改参数的默认值,然后在命令行中指定不同的参数值来运行脚本。例如:
```
python your_script.py --device cuda:0 --num-classes 5 --data-path /path/to/dataset --weights-path /path/to/weights.pth --batch_size 4
```
这样就可以传入不同的参数值来运行脚本了。
请帮我翻译每一句代码:def parse_opt(): parser = argparse.ArgumentParser() parser.add_argument('--weights', nargs='+', type=str, default='D://Net//pytorch//yolov5-master//yolov5-master//runs//train//exp3//weights//best.pt', help='model path or triton URL') parser.add_argument('--source', type=str, default=ROOT / 'data/images', help='file/dir/URL/glob/screen/0(webcam)') parser.add_argument('--data', type=str, default=ROOT / 'data/coco128.yaml', help='(optional) dataset.yaml path') parser.add_argument('--imgsz', '--img', '--img-size', nargs='+', type=int, default=[480], help='inference size h,w') parser.add_argument('--conf-thres', type=float, default=0.25, help='confidence threshold') parser.add_argument('--iou-thres', type=float, default=0.45, help='NMS IoU threshold') parser.add_argument('--max-det', type=int, default=1000, help='maximum detections per image') parser.add_argument('--device', default='', help='cuda device, i.e. 0 or 0,1,2,3 or cpu') parser.add_argument('--view-img', action='store_true', help='show results') parser.add_argument('--save-txt', action='store_true', help='save results to *.txt') parser.add_argument('--save-conf', action='store_true', help='save confidences in --save-txt labels') parser.add_argument('--save-crop', action='store_true', help='save cropped prediction boxes') parser.add_argument('--nosave', action='store_true', help='do not save images/videos') parser.add_argument('--classes', nargs='+', type=int, help='filter by class: --classes 0, or --classes 0 2 3') parser.add_argument('--agnostic-nms', action='store_true', help='class-agnostic NMS') parser.add_argument('--augment', action='store_true', help='augmented inference') parser.add_argument('--visualize', action='store_true', help='visualize features')
def parse_opt():
# 定义 argparse 解析器
parser = argparse.ArgumentParser()
# 添加命令行参数
parser.add_argument('--weights', nargs='+', type=str, default='D://Net//pytorch//yolov5-master//yolov5-master//runs//train//exp3//weights//best.pt', help='model path or triton URL')
parser.add_argument('--source', type=str, default=ROOT / 'data/images', help='file/dir/URL/glob/screen/0(webcam)')
parser.add_argument('--data', type=str, default=ROOT / 'data/coco128.yaml', help='(optional) dataset.yaml path')
parser.add_argument('--imgsz', '--img', '--img-size', nargs='+', type=int, default=[480], help='inference size h,w')
parser.add_argument('--conf-thres', type=float, default=0.25, help='confidence threshold')
parser.add_argument('--iou-thres', type=float, default=0.45, help='NMS IoU threshold')
parser.add_argument('--max-det', type=int, default=1000, help='maximum detections per image')
parser.add_argument('--device', default='', help='cuda device, i.e. 0 or 0,1,2,3 or cpu')
parser.add_argument('--view-img', action='store_true', help='show results')
parser.add_argument('--save-txt', action='store_true', help='save results to *.txt')
parser.add_argument('--save-conf', action='store_true', help='save confidences in --save-txt labels')
parser.add_argument('--save-crop', action='store_true', help='save cropped prediction boxes')
parser.add_argument('--nosave', action='store_true', help='do not save images/videos')
parser.add_argument('--classes', nargs='+', type=int, help='filter by class: --classes 0, or --classes 0 2 3')
parser.add_argument('--agnostic-nms', action='store_true', help='class-agnostic NMS')
parser.add_argument('--augment', action='store_true', help='augmented inference')
parser.add_argument('--visualize', action='store_true', help='visualize features')
# 返回解析器
return parser
相关推荐
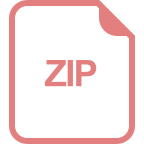
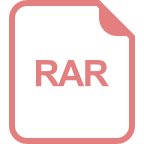











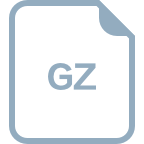