modbus c语言源码
时间: 2023-07-28 12:07:18 浏览: 55
以下是一个简单的使用C语言实现Modbus协议的源代码示例,供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <string.h>
#define SLAVE_ADDRESS 0x01
/* Modbus function codes */
#define READ_COILS 0x01
#define READ_DISCRETE_INPUTS 0x02
#define READ_HOLDING_REGISTERS 0x03
#define READ_INPUT_REGISTERS 0x04
#define WRITE_SINGLE_COIL 0x05
#define WRITE_SINGLE_REGISTER 0x06
#define WRITE_MULTIPLE_COILS 0x0F
#define WRITE_MULTIPLE_REGISTERS 0x10
/* Modbus exception codes */
#define EXCEPTION_ILLEGAL_FUNCTION 0x01
#define EXCEPTION_ILLEGAL_DATA_ADDRESS 0x02
#define EXCEPTION_ILLEGAL_DATA_VALUE 0x03
#define EXCEPTION_SLAVE_DEVICE_FAILURE 0x04
#define EXCEPTION_ACKNOWLEDGE 0x05
#define EXCEPTION_SLAVE_DEVICE_BUSY 0x06
#define EXCEPTION_MEMORY_PARITY_ERROR 0x08
#define EXCEPTION_GATEWAY_PATH_UNAVAILABLE 0x0A
#define EXCEPTION_GATEWAY_TARGET_DEVICE_FAILED_TO_RESPOND 0x0B
/* Modbus TCP/IP frame format */
typedef struct {
uint16_t transaction_identifier;
uint16_t protocol_identifier;
uint16_t length;
uint8_t unit_identifier;
uint8_t function_code;
uint8_t data[];
} modbus_frame_t;
/* Modbus response frame format */
typedef struct {
uint16_t transaction_identifier;
uint16_t protocol_identifier;
uint16_t length;
uint8_t unit_identifier;
uint8_t function_code;
uint8_t byte_count;
uint8_t data[];
} modbus_response_t;
/* Modbus exception response frame format */
typedef struct {
uint16_t transaction_identifier;
uint16_t protocol_identifier;
uint16_t length;
uint8_t unit_identifier;
uint8_t function_code;
uint8_t exception_code;
} modbus_exception_t;
/* Calculate Modbus CRC */
uint16_t modbus_crc(uint8_t *data, uint16_t length) {
uint16_t crc = 0xFFFF;
uint8_t i, j;
for (i = 0; i < length; i++) {
crc ^= data[i];
for (j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
return crc;
}
/* Send Modbus request and receive response */
int modbus_request(uint8_t *request, uint16_t request_length, uint8_t *response, uint16_t *response_length) {
/* TODO: Implement Modbus request and response handling */
return 0;
}
/* Read multiple holding registers */
int modbus_read_holding_registers(uint16_t register_address, uint16_t register_count, uint16_t *register_values) {
uint8_t request[256];
uint16_t request_length;
uint8_t response[256];
uint16_t response_length;
/* Build Modbus request */
request_length = 0;
request[request_length++] = SLAVE_ADDRESS;
request[request_length++] = READ_HOLDING_REGISTERS;
request[request_length++] = (register_address >> 8) & 0xFF;
request[request_length++] = register_address & 0xFF;
request[request_length++] = (register_count >> 8) & 0xFF;
request[request_length++] = register_count & 0xFF;
/* Calculate Modbus CRC */
uint16_t crc = modbus_crc(request, request_length);
request[request_length++] = crc & 0xFF;
request[request_length++] = (crc >> 8) & 0xFF;
/* Send Modbus request and receive response */
if (modbus_request(request, request_length, response, &response_length) != 0) {
printf("Error: Modbus request failed!\n");
return -1;
}
/* Parse Modbus response */
modbus_response_t *modbus_response = (modbus_response_t *) response;
if (modbus_response->unit_identifier != SLAVE_ADDRESS) {
printf("Error: Invalid Modbus slave address!\n");
return -1;
}
if (modbus_response->function_code == READ_HOLDING_REGISTERS + 0x80) {
modbus_exception_t *modbus_exception = (modbus_exception_t *) response;
printf("Error: Modbus exception code %d!\n", modbus_exception->exception_code);
return -1;
}
if (modbus_response->function_code != READ_HOLDING_REGISTERS) {
printf("Error: Invalid Modbus function code!\n");
return -1;
}
if (modbus_response->byte_count != register_count * 2) {
printf("Error: Invalid Modbus byte count!\n");
return -1;
}
memcpy(register_values, modbus_response->data, register_count * 2);
return 0;
}
/* Main function */
int main(int argc, char **argv) {
/* Read holding registers */
uint16_t register_values[16];
if (modbus_read_holding_registers(0x0000, 16, register_values) != 0) {
printf("Error: Failed to read Modbus holding registers!\n");
return -1;
}
for (int i = 0; i < 16; i++) {
printf("Register %d: %d\n", i, register_values[i]);
}
return 0;
}
```
请注意,这个示例仅仅是一个简单的模板,实际应用中需要根据具体情况进行修改和完善。同时,还需要实现Modbus协议的物理层和传输层,例如串口通信或TCP/IP通信。
相关推荐
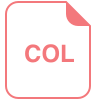













