编写用c语言的栈将十进制转换为十六进制的代码
时间: 2023-08-31 17:42:15 浏览: 47
### 回答1:
好的,下面是用C语言编写栈将十进制转换为十六进制的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_STACK_SIZE 100
typedef struct {
int top;
int data[MAX_STACK_SIZE];
} Stack;
/* 初始化栈 */
void init(Stack *s) {
s->top = -1;
}
/* 将元素压入栈中 */
void push(Stack *s, int value) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Stack is full.\n");
exit(1);
} else {
s->data[++s->top] = value;
}
}
/* 从栈中弹出元素 */
int pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty.\n");
exit(1);
} else {
return s->data[s->top--];
}
}
/* 十进制转十六进制 */
void decimalToHex(int decimal) {
Stack s;
init(&s);
int temp;
while (decimal != 0) {
temp = decimal % 16;
push(&s, temp);
decimal = decimal / 16;
}
int hex;
printf("The hexadecimal value is: ");
while (s.top != -1) {
hex = pop(&s);
if (hex < 10) {
printf("%d", hex);
} else {
printf("%c", hex - 10 + 'A');
}
}
printf("\n");
}
int main() {
int decimal;
printf("Please enter a decimal number: ");
scanf("%d", &decimal);
decimalToHex(decimal);
return 0;
}
```
### 回答2:
以下是用C语言编写的将十进制数转换为十六进制的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义栈的结构
typedef struct {
int top;
int items[MAX_SIZE];
} Stack;
// 初始化栈
void initialize(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return (s->top == -1);
}
// 判断栈是否已满
int isFull(Stack *s) {
return (s->top == MAX_SIZE - 1);
}
// 入栈操作
void push(Stack *s, int value) {
if (isFull(s)) {
printf("栈已满,无法入栈\n");
return;
}
s->items[++(s->top)] = value;
}
// 出栈操作
int pop(Stack *s) {
if (isEmpty(s)) {
printf("栈为空,无法出栈\n");
return -1;
}
return s->items[(s->top)--];
}
// 十进制转换为十六进制函数
void decimalToHexadecimal(int decimal) {
Stack s;
initialize(&s);
// 处理特殊情况
if (decimal == 0) {
printf("十六进制数为 0\n");
return;
}
// 将十进制数转换为十六进制,并入栈
while (decimal != 0) {
int remainder = decimal % 16;
push(&s, remainder);
decimal /= 16;
}
// 出栈并打印栈中的元素即为十六进制数
printf("十六进制数为 ");
while (!isEmpty(&s)) {
int value = pop(&s);
if (value < 10) {
printf("%d", value);
} else {
printf("%c", value + 55); // A~F对应的 ASCII 值为65~70,故需加55
}
}
printf("\n");
}
int main() {
int decimal;
printf("请输入一个十进制数: ");
scanf("%d", &decimal);
decimalToHexadecimal(decimal);
return 0;
}
```
这段代码用到了栈的数据结构,通过模拟将十进制数转换为十六进制的过程实现。
### 回答3:
以下是用C语言编写的将十进制转换为十六进制的代码:
```c
#include <stdio.h>
#include <stdlib.h>
void convertToHex(int decimal) {
int quotient, remainder;
int i = 0;
char hex[100];
if (decimal == 0) {
printf("0");
return;
}
while (decimal != 0) {
quotient = decimal / 16;
remainder = decimal % 16;
if (remainder < 10) {
hex[i] = remainder + 48; // 转换为对应的ASCII码
} else {
hex[i] = remainder + 55; // 转换为对应的ASCII码
}
decimal = quotient;
i++;
}
for (int j = i - 1; j >= 0; j--) {
printf("%c", hex[j]);
}
}
int main() {
int decimal;
printf("输入一个十进制数:");
scanf("%d", &decimal);
printf("转换为十六进制:");
convertToHex(decimal);
return 0;
}
```
这段代码中,`convertToHex`函数接收一个十进制数作为参数,并将其转换为十六进制,然后通过循环将得到的十六进制数字逐个输出。在`main`函数中,用户需要输入一个十进制数,然后调用`convertToHex`函数来进行转换并输出结果。
相关推荐
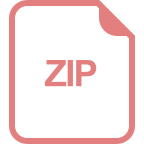














