设计算法,实现两个顺序表集合A和B的并集操作(并集 中要去掉集合A和B中的相同元素)。 •思路: •void UnionL(SqList * La, SqList * Lb) •遍历B中的每个元素,判断当前元素是否存在A中,若不存 在,则插入到A的末尾,最后得到的集合A就是并集。用c语言写一段完整的代码
时间: 2024-02-13 08:59:36 浏览: 92
以下是用C语言实现两个顺序表集合A和B的并集操作的完整代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int length;
} SqList;
void InitList(SqList *L) {
L->length = 0;
}
void InsertElem(SqList *L, int e) {
if (L->length == MAXSIZE) {
printf("List is full!\n");
return;
}
L->data[L->length] = e;
L->length++;
}
void UnionL(SqList *La, SqList *Lb) {
int i, j;
for (i = 0; i < Lb->length; i++) {
for (j = 0; j < La->length; j++) {
if (Lb->data[i] == La->data[j]) {
break;
}
}
if (j == La->length) {
InsertElem(La, Lb->data[i]);
}
}
}
void PrintList(SqList L) {
int i;
for (i = 0; i < L.length; i++) {
printf("%d ", L.data[i]);
}
printf("\n");
}
int main() {
SqList La, Lb;
InitList(&La);
InitList(&Lb);
InsertElem(&La, 1);
InsertElem(&La, 2);
InsertElem(&La, 3);
InsertElem(&Lb, 2);
InsertElem(&Lb, 4);
InsertElem(&Lb, 5);
UnionL(&La, &Lb);
printf("La: ");
PrintList(La);
printf("Lb: ");
PrintList(Lb);
return 0;
}
```
程序中,我们定义了一个 `SqList` 结构体,其中 `data` 数组存放顺序表中的元素, `length` 表示顺序表的长度。`InitList` 函数用于初始化顺序表, `InsertElem` 函数用于在顺序表的末尾插入元素。`UnionL` 函数实现了两个顺序表的并集操作,其中使用了两个循环,第一个循环遍历集合B中的每个元素,第二个循环遍历集合A中的每个元素,判断是否存在相同元素,若不存在,则将该元素插入到集合A的末尾。 `PrintList` 函数用于输出顺序表中的元素。在 `main` 函数中,我们初始化两个顺序表La和Lb,向La中插入元素1、2、3,向Lb中插入元素2、4、5,然后调用 `UnionL` 函数对两个顺序表求并集,并输出结果。
阅读全文
相关推荐
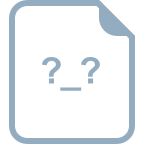
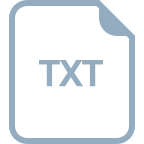














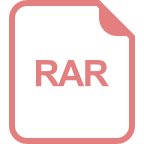