基于python写一个程序,实现电阻层析成像的逆问题求解与图像重构算法
时间: 2024-10-09 21:01:18 浏览: 74
电阻层析成像(Electrical Resistivity Tomography, ERT)是一种利用地下导电性的差异来形成地球内部结构模型的技术。在Python中,你可以使用一些科学计算库如NumPy、SciPy和Matplotlib来进行数学建模和图像处理。
为了编写一个简单的ERT逆问题求解和图像重构程序,你可以按照以下步骤:
1. **导入所需库**:
```python
import numpy as np
from scipy.optimize import least_squares
import matplotlib.pyplot as plt
```
2. **模拟数据生成**:
- 定义电阻率分布(通常是一个二维数组)
- 根据分布创建电压测量数据(考虑到实际实验中的电流注入和测量)
```python
def forward_model(resistivity_map, current_injection, measurement_positions):
# 这里是一个简化版的模型,真实情况下需要考虑导体几何和电势计算
potential = ...
return potential
# 示例电阻率分布
resistivity = np.random.rand(50, 50)
currents = np.random.randn(10, 2) # 10个电流源位置
measurements = ... # 模拟的电压测量结果
```
3. **逆问题求解(最小二乘法)**:
使用`least_squares`函数求解电阻率分布,使得模型预测的电压最接近实际测量值。
```python
def objective_function(resistivity_map, data, model):
return forward_model(resistivity_map, currents, measurements) - data
resistivity_recovered, _ = least_squares(objective_function, resistivity, args=(measurements, forward_model))
```
4. **图像重构和可视化**:
重建后的电阻率图并显示。
```python
reconstructed_image = resistivity_recovered.reshape((50, 50))
plt.imshow(reconstructed_image, cmap='gray')
plt.title('Reconstructed Resitivity Image')
plt.show()
```
阅读全文
相关推荐
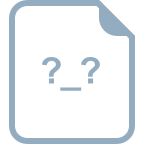
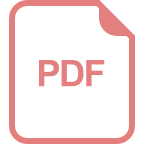
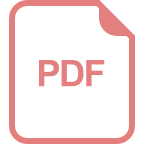
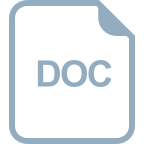
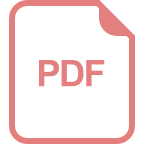
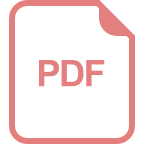
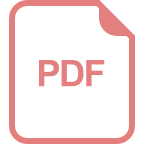
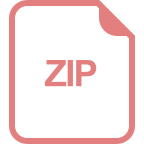
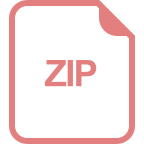
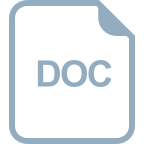
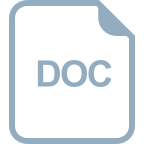
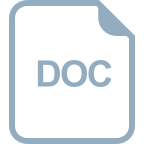
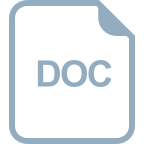
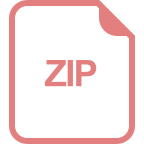