module.exports = { configureWebpack: { devServer: { proxy: { '/api': { target: 'http://127.0.0.1:8088',// flowable // target: 'http://127.0.0.1:8089', // activiti changeOrigin: true, pathRewrite: { '^/api': '/' } } } } } }
时间: 2024-02-14 10:21:43 浏览: 26
这段代码是用于配置 Vue.js 项目的 webpack 打包工具。其中,使用了 devServer 来启动开发服务器,并且设置了代理规则,将以 /api 开头的请求转发到 target 指定的地址上。这个配置的意义在于,当我们在开发 Vue.js 项目时,后端服务可能运行在另外一个端口或者另外一台机器上,使用代理可以让前端代码直接请求本地的 devServer,而不需要手动切换接口地址。需要注意的是,这里的代理规则是针对 flowable 服务的,如果需要代理 activiti 服务,需要将 target 的值修改为相应的地址。
相关问题
解释代码:module.exports = { devServer: { proxy: "http://localhost:8081" },
这段代码是一个 Node.js 模块的导出语句,其中 module.exports 是 Node.js 中用于导出模块的对象,它可以包含任何类型的数据,包括函数、对象、数组等。在这里,它导出了一个对象,其中包含一个 devServer 属性,它的值是一个对象,其中包含一个 proxy 属性,它的值是一个字符串 "http://localhost:8081",表示将所有请求代理到本地的 8081 端口。这段代码通常用于 Vue.js 或 React.js 等前端框架的开发中,用于解决跨域请求的问题。
const { defineConfig } = require('@vue/cli-service') module.exports = defineConfig({ transpileDependencies: true }) module.exports = { devServer: { proxy: { '/api': { target: 'http://localhost:3000', changeOrigin: true, pathRewrite: { '^/api': '' } } } } };
这段代码有一个问题,因为你使用了两次`module.exports`来导出配置,只有最后一次导出的配置会生效。你需要将两个配置合并到一个对象中,然后再进行导出。
你可以使用`Object.assign()`方法或者展开运算符`...`来合并两个对象。修改代码如下所示:
```javascript
const { defineConfig } = require('@vue/cli-service')
module.exports = defineConfig({
transpileDependencies: true,
devServer: {
proxy: {
'/api': {
target: 'http://localhost:3000',
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
}
});
```
这样,你的配置中既包含了 Vue CLI 的配置(transpileDependencies),也包含了 devServer 的配置(proxy)。
希望对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
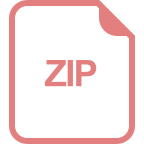
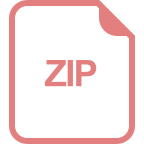
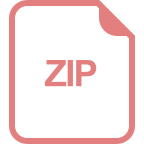













