docx文件:import re # 读取文件内容 with open("example_output.txt", "r", encoding="utf-8") as f: content = f.read() # 使用正则表达式去除重复 pattern = r'^(\d+)\.\s―.*\[答案\]\s(\w).*$' result = {} for line in content.split('\n'): match = re.match(pattern, line) if match: q_num = match.group(1) answer = match.group(2) result[q_num] = answer # 将去重后的内容写入新文件 with open("example_output_no_duplicate.txt", "w", encoding="utf-8") as f: for q_num, answer in result.items(): f.write(f"{q_num}. ―___________________ [答案] {answer}\n")
时间: 2024-01-02 14:04:22 浏览: 20
对于docx文件,可以使用`docx2txt`库来读取文件内容。以下是修改后的代码:
```python
import re
import docx2txt
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式去除重复
pattern = r'^(\d+)\.\s―.*\[答案\]\s(\w).*$'
result = {}
for line in text.split('\n'):
match = re.match(pattern, line)
if match:
q_num = match.group(1)
answer = match.group(2)
result[q_num] = answer
# 将去重后的内容写入新文件
with open("example_output_no_duplicate.txt", "w", encoding="utf-8") as f:
for q_num, answer in result.items():
f.write(f"{q_num}. ―___________________ [答案] {answer}\n")
```
该代码通过`docx2txt.process()`函数读取docx文件内容,并使用正则表达式去除重复。最后将去重后的内容写入新文件。
相关问题
import docx2txt import re # 读取docx文件内容 text = docx2txt.process("example.docx") # 使用正则表达式去重 pattern = r'^(?:(\d+).\s)?―.\n.?[答案]\s*(\w+)\b.*$' # 将去重后的内容写入新的文本文件 with open("example_output.txt", "w", encoding="utf-8") as f: f.write('\n'.join(result.keys()))
这段代码中使用了正则表达式去重,但是没有将匹配到的结果保存下来。可以在代码中添加如下部分,将匹配到的结果保存到一个字典中:
import docx2txt
import re
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式去重
pattern = r'^(?:(\d+).\s)?―.\n.?[答案]\s*(\w+)\b.*$'
result = {}
for line in text.split('\n'):
match = re.match(pattern, line)
if match:
q_num = match.group(1)
answer = match.group(2)
result[q_num] = answer
# 将去重后的内容写入新的文本文件
with open("example_output.txt", "w", encoding="utf-8") as f:
f.write('\n'.join(result.keys()))
优化代码:import docx2txt import re # 读取docx文件内容 text = docx2txt.process("example.docx") # 将内容写入文本文件 with open("example.txt", "w", encoding="utf-8") as f: f.write(text) # 读取文本文件内容 with open("example.txt", "r", encoding="utf-8") as f: lines = f.readlines() # 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容并去重 pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+' result = re.findall(pattern, text) result = list(set(result)) print(result) # 将去重后的内容重新写入文本文件 with open("example.txt", "w", encoding="utf-8") as f: f.writelines(result)
可以考虑将代码优化为一次性读取docx文件,并且将匹配结果直接写入新的文本文件,避免先写入一次文本文件再读取一次的操作。代码如下:
```
import docx2txt
import re
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容并去重
pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+'
result = list(set(re.findall(pattern, text)))
# 将去重后的内容写入新的文本文件
with open("example_output.txt", "w", encoding="utf-8") as f:
f.write('\n'.join(result))
```
这样可以避免不必要的IO操作,并且将结果直接写入新的文本文件。
相关推荐
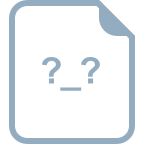
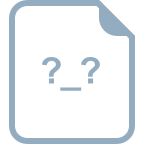












