Android。字符串去重
时间: 2023-12-29 14:06:27 浏览: 28
可以通过使用 HashSet 来去除字符串中的重复项。HashSet 是一种无序的、不允许重复元素的集合。可以将字符串转换为字符数组,然后使用 HashSet 对字符数组进行去重,最后将结果转换回字符串。
以下是一个示例代码:
```
String str = "hello world";
char[] charArray = str.toCharArray();
Set<Character> charSet = new HashSet<>();
for (char c : charArray) {
charSet.add(c);
}
StringBuilder sb = new StringBuilder();
for (Character c : charSet) {
sb.append(c);
}
String result = sb.toString();
```
在上面的代码中,首先将字符串转换为字符数组。然后使用 HashSet 对字符数组进行去重,最后将结果转换回字符串。
注意,上面的代码只是对一个字符串中的字符进行去重,如果需要对多个字符串进行去重,可以使用类似的方法将它们合并为一个字符串后再进行去重。
相关问题
qt 字符串去重
你可以使用 Qt 的 QSet 类来实现字符串去重。首先,将字符串分割成单个字符,然后将这些字符添加到 QSet 中,由于 QSet 自身的特性,会自动去除重复的字符。最后,将 QSet 中的字符再组合成字符串即可。
以下是一个示例代码:
```cpp
QString str = "hello world";
QSet<QString> charSet;
for (int i = 0; i < str.length(); i++) {
charSet.insert(QString(str[i]));
}
QString result;
foreach(QString s, charSet) {
result += s;
}
qDebug() << result; // 输出 "helo wrd"
```
其中,`charSet.insert(QString(str[i]))` 将一个字符转换为字符串并添加到 QSet 中。`foreach` 循环用于遍历 QSet 中的所有字符,并将它们拼接成一个新的字符串。
Java字符串去重
可以使用Set数据结构来实现Java字符串去重,具体代码如下:
```java
String str = "aaabbbccc";
Set<Character> set = new HashSet<>();
for (int i = 0; i < str.length(); i++) {
set.add(str.charAt(i));
}
StringBuilder sb = new StringBuilder();
for (Character c : set) {
sb.append(c);
}
String result = sb.toString(); // 去重后的字符串
```
上述代码中,首先将字符串中的每一个字符添加到Set中,由于Set会自动去重,所以最后Set中只会保留不重复的字符。然后再将Set中的字符按顺序拼接起来,即可得到去重后的字符串。
相关推荐
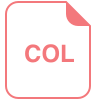
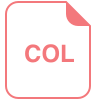
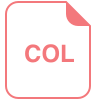
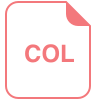
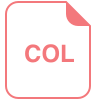







