如何用C++编程实现下列两项功能:1.利用Iseek空洞文件原理,来完成对文件的分片拷贝 2.实现一个cp命令,将a.txt文件内的内容拷贝到b.txt文件中
时间: 2024-04-12 08:32:13 浏览: 23
对于第一个功能,利用Iseek空洞文件原理来完成对文件的分片拷贝,可以按照以下步骤进行:
1. 打开源文件和目标文件,使用`open`函数。
```cpp
#include <fcntl.h>
#include <unistd.h>
int srcFile = open("source.txt", O_RDONLY);
int destFile = open("destination.txt", O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
```
2. 使用`lseek`函数将目标文件扩展到与源文件相同大小。
```cpp
off_t fileSize = lseek(srcFile, 0, SEEK_END);
lseek(destFile, fileSize - 1, SEEK_SET);
write(destFile, "", 1);
```
3. 使用`sendfile`函数进行文件拷贝。
```cpp
off_t offset = 0;
ssize_t bytesSent = 0;
while (offset < fileSize) {
bytesSent = sendfile(destFile, srcFile, &offset, fileSize - offset);
}
```
4. 关闭文件。
```cpp
close(srcFile);
close(destFile);
```
对于第二个功能,实现一个`cp`命令将`a.txt`文件内的内容拷贝到`b.txt`文件中,可以使用以下方法:
1. 打开源文件和目标文件,使用`ifstream`和`ofstream`。
```cpp
#include <fstream>
std::ifstream source("a.txt", std::ios::binary);
std::ofstream dest("b.txt", std::ios::binary);
```
2. 使用`istreambuf_iterator`和`ostreambuf_iterator`进行文件内容拷贝。
```cpp
std::copy(std::istreambuf_iterator<char>(source), std::istreambuf_iterator<char>(),
std::ostreambuf_iterator<char>(dest));
```
3. 关闭文件。
```cpp
source.close();
dest.close();
```
这样就可以实现将`a.txt`文件的内容拷贝到`b.txt`文件中。
相关推荐
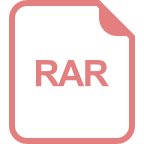
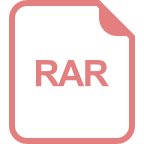
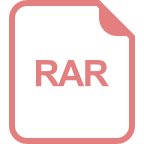
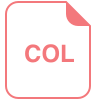
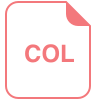
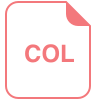
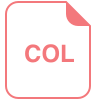
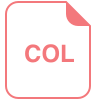









