gstreamer pipeline 状态监控, c代码例子
时间: 2023-06-07 09:08:58 浏览: 149
可以提供一个基于GStreamer的状态监控的C代码示例:
```c
#include <gst/gst.h>
static void on_status_changed(GstBus *bus, GstMessage *message, gpointer user_data) {
GError *error = NULL;
gchar *debug_info = NULL;
GstElement *element = GST_ELEMENT(message->src);
const GstStructure *structure = gst_message_get_structure(message);
const gchar *status;
status = gst_structure_get_string(structure, "status");
gst_message_parse_error(message, &error, &debug_info);
g_print("Status: %s\n", status);
if (error != NULL) {
g_print("Error: %s\n", error->message);
g_error_free(error);
}
if (debug_info != NULL) {
g_print("Debug info: %s\n", debug_info);
g_free(debug_info);
}
}
int main(int argc, char *argv[]) {
GstElement *pipeline = NULL;
GstBus *bus = NULL;
GstCaps *caps = NULL;
GstStateChangeReturn ret;
gst_init(&argc, &argv);
// Create pipeline
pipeline = gst_pipeline_new("test-pipeline");
// Add elements to pipeline
GstElement *src = gst_element_factory_make("videotestsrc", "src");
GstElement *sink = gst_element_factory_make("autovideosink", "sink");
gst_bin_add_many(GST_BIN(pipeline), src, sink, NULL);
// Link elements
if (!gst_element_link(src, sink)) {
g_printerr("Failed to link elements\n");
gst_object_unref(pipeline);
return -1;
}
// Set pipeline to playing state
ret = gst_element_set_state(pipeline, GST_STATE_PLAYING);
if (ret == GST_STATE_CHANGE_FAILURE) {
g_printerr("Failed to set pipeline to playing state\n");
gst_object_unref(pipeline);
return -1;
}
// Get a bus and connect to the source element's bus
bus = gst_element_get_bus(pipeline);
gst_bus_add_watch(bus, on_status_changed, NULL);
// Run main loop
GstMessage *message = gst_bus_timed_pop_filtered(bus, GST_CLOCK_TIME_NONE, GST_MESSAGE_ERROR | GST_MESSAGE_EOS);
if (message != NULL) {
gst_message_unref(message);
}
// Free resources
gst_object_unref(bus);
gst_element_set_state(pipeline, GST_STATE_NULL);
gst_object_unref(pipeline);
return 0;
}
```
该示例中,我们创建一个简单的 GStreamer pipeline,由 videotestsrc 元件产生视频数据,再经由 autovideosink 元件输出到屏幕上。我们向 pipeline 的总线(bus)注册了 on_status_changed 回调函数,在该回调函数中,我们可以根据状态信息(status)判断 pipeline 的运行状态,并在发生错误时输出错误信息以及调试信息。
阅读全文
相关推荐
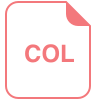
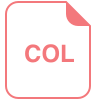
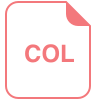
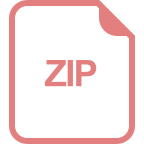
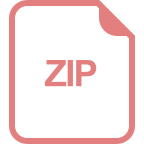
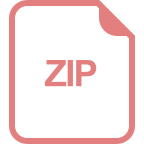
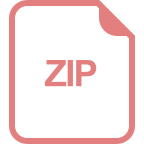
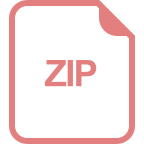
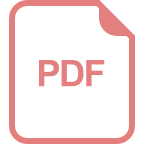
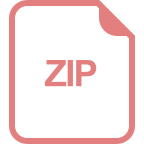