pygame立方体
时间: 2023-07-10 14:15:32 浏览: 34
好的,我可以为您提供一个简单的 Pygame 立方体的代码示例。首先,你需要安装 Pygame 库,然后可以按照以下步骤进行操作:
1. 导入 Pygame 库和 math 库
```python
import pygame
import math
```
2. 初始化 Pygame
```python
pygame.init()
```
3. 创建一个窗口并设置其大小
```python
screen = pygame.display.set_mode((600, 600))
```
4. 定义立方体的初始位置和大小
```python
cube_size = 100
cube_pos = [300, 300, 0]
```
5. 定义立方体的顶点坐标
```python
vertices = [
[-1, 1, -1],
[1, 1, -1],
[1, -1, -1],
[-1, -1, -1],
[-1, 1, 1],
[1, 1, 1],
[1, -1, 1],
[-1, -1, 1]
]
```
6. 定义立方体的面
```python
faces = [
[0, 1, 2, 3],
[1, 5, 6, 2],
[5, 4, 7, 6],
[4, 0, 3, 7],
[0, 4, 5, 1],
[3, 2, 6, 7]
]
```
7. 定义一个函数,用于将立方体的顶点坐标旋转到指定的角度
```python
def rotate_cube(angle_x, angle_y, angle_z):
rotation_x = [
[1, 0, 0],
[0, math.cos(angle_x), -math.sin(angle_x)],
[0, math.sin(angle_x), math.cos(angle_x)]
]
rotation_y = [
[math.cos(angle_y), 0, math.sin(angle_y)],
[0, 1, 0],
[-math.sin(angle_y), 0, math.cos(angle_y)]
]
rotation_z = [
[math.cos(angle_z), -math.sin(angle_z), 0],
[math.sin(angle_z), math.cos(angle_z), 0],
[0, 0, 1]
]
rotation_matrix = math.matrix(rotation_x) * math.matrix(rotation_y) * math.matrix(rotation_z)
rotated_vertices = []
for vertex in vertices:
rotated_vertex = math.matrix(vertex) * rotation_matrix
rotated_vertices.append(rotated_vertex)
return rotated_vertices
```
8. 在主循环中更新立方体的位置和角度,并绘制立方体
```python
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
angle_x += 0.01
angle_y += 0.01
angle_z += 0.01
rotated_vertices = rotate_cube(angle_x, angle_y, angle_z)
for face in faces:
point_list = []
for vertex_index in face:
vertex = rotated_vertices[vertex_index]
x = int(vertex[0] * cube_size / (vertex[2] + cube_size) + cube_pos[0])
y = int(vertex[1] * cube_size / (vertex[2] + cube_size) + cube_pos[1])
point_list.append([x, y])
pygame.draw.polygon(screen, (0, 0, 0), point_list)
pygame.display.update()
```
这是一个简单的 Pygame 立方体的代码示例。当你运行这个程序时,你将看到一个旋转的立方体。你可以根据自己的需要进行修改和调整。
相关推荐
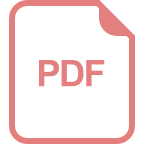














