按键控制12位数码管代码 stm32
时间: 2024-05-10 08:16:16 浏览: 11
首先需要确定使用的是哪一种12位数码管,因为不同的数码管使用不同的驱动方式。常见的有共阳数码管和共阴数码管。
以共阳数码管为例,可以使用以下代码进行键盘输入控制:
```c
#include "stm32f10x.h"
int main(void)
{
// 使能外设时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置GPIO引脚为输入模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置GPIO引脚为输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 |
GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7 |
GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
int num = 0; // 初始数字
int i;
while (1)
{
for (i = 0; i < 4; i++)
{
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0 << i) == 0) // 检测到按键按下
{
num++; // 数字加1
if (num >= 10000) // 数字循环
{
num = 0;
}
}
}
// 显示数字
int digit[12] = {0}; // 数码管状态数组,共阳数码管为0表示点亮
digit[0] = num % 10;
digit[1] = num / 10 % 10;
digit[2] = num / 100 % 10;
digit[3] = num / 1000 % 10;
// 输出到数码管
for (i = 0; i < 12; i++)
{
if (digit[i] == 0)
{
GPIO_SetBits(GPIOB, GPIO_Pin_0 << i);
}
else
{
GPIO_ResetBits(GPIOB, GPIO_Pin_0 << i);
}
}
}
}
```
这段代码使用了GPIOA的0~3引脚作为按键输入,GPIOB的0~11引脚作为数码管输出。按键按下后数字加1,数字循环在0~9999之间。将数字转换成数码管状态数组,根据数组输出到数码管。其中GPIO_SetBits和GPIO_ResetBits函数用于设置引脚输出高电平或低电平,从而控制数码管点亮。
相关推荐
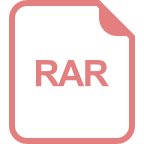
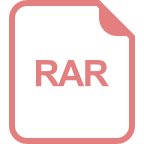














