定义一个基类“Role”,类内有数据成员姓名,年龄,性别,有对各数据成员进行读写的函数getXXX()和setXXX(),有一个虚函数display(),该函数显示姓名,年龄,性别等数据成员的值,同时类内至少定义两个构造函数。 从Role类派生出一个“Employee”类,该类具有Role类的所有成员(构造函数除外),并扩展数据成员ID (职工编号)和salary(薪资)。同样要有至少两个构造方法,并重新定义display()函数。 主函数内定义基类指针和派生类对象,并使基类指针指向派生类对象,通过该基类指针访问虚函数display()。
时间: 2024-03-19 09:45:49 浏览: 50
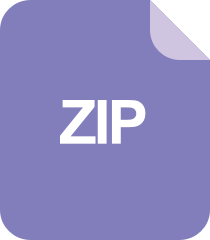
C抽象基类和纯虚成员函数-C教程共3页.pdf.zip
好的,这是一个关于C++面向对象的问题,下面是我给你的答案:
```c++
#include<iostream>
#include<string>
using namespace std;
class Role {
private:
string name;
int age;
string gender;
public:
Role(string name, int age, string gender) {
this->name = name;
this->age = age;
this->gender = gender;
}
Role() {
this->name = "";
this->age = 0;
this->gender = "";
}
void setName(string name) {
this->name = name;
}
string getName() {
return this->name;
}
void setAge(int age) {
this->age = age;
}
int getAge() {
return this->age;
}
void setGender(string gender) {
this->gender = gender;
}
string getGender() {
return this->gender;
}
virtual void display() {
cout << "Name: " << this->name << endl;
cout << "Age: " << this->age << endl;
cout << "Gender: " << this->gender << endl;
}
};
class Employee : public Role {
private:
string ID;
double salary;
public:
Employee(string name, int age, string gender, string ID, double salary) : Role(name, age, gender) {
this->ID = ID;
this->salary = salary;
}
Employee() : Role() {
this->ID = "";
this->salary = 0;
}
void setID(string ID) {
this->ID = ID;
}
string getID() {
return this->ID;
}
void setSalary(double salary) {
this->salary = salary;
}
double getSalary() {
return this->salary;
}
void display() {
Role::display();
cout << "ID: " << this->ID << endl;
cout << "Salary: " << this->salary << endl;
}
};
int main() {
Role* r = new Employee("Tom", 25, "Male", "001", 5000);
r->display();
return 0;
}
```
这段代码定义了一个基类Role和一个派生类Employee。其中,Role类有姓名、年龄、性别三个数据成员和对应的读写函数,以及一个虚函数display();Employee类继承了Role类,并在其基础上增加了ID和salary两个数据成员和对应的读写函数,同时重新定义了display()函数。
主函数中定义了Role类的指针r,并将其指向Employee类的对象,然后通过r调用display()函数,由于display()是虚函数,所以会调用Employee类中重新定义的display()函数,输出Employee类的所有数据成员。
阅读全文
相关推荐
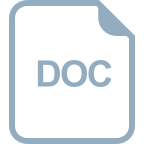
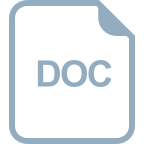




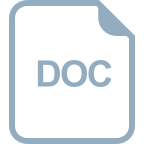
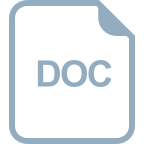
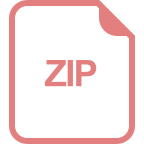
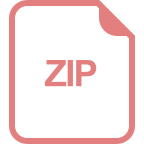
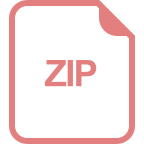
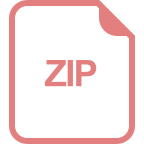
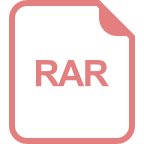
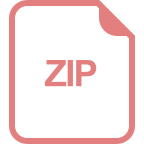
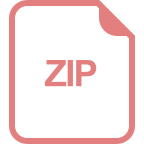
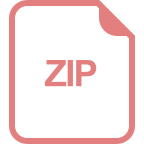
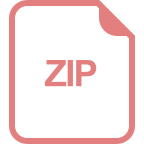