springboot mybatis 在表A表B表C中修改查询条件查出来的编码并且是唯一 的接口怎么写
时间: 2024-03-27 07:39:20 浏览: 24
首先,在 Spring Boot 中使用 MyBatis,需要在 pom.xml 中引入相应的依赖:
```xml
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
```
其次,需要在 application.properties 文件中配置 MyBatis 的相关参数,如数据源、Mapper 文件的位置等:
```properties
# 数据源配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# MyBatis 配置
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.type-aliases-package=com.example.entity
```
其中,`mybatis.mapper-locations` 指定了 Mapper 文件的位置,`mybatis.type-aliases-package` 指定了实体类的包名。
接下来,我们可以在 Mapper 文件中编写查询语句,例如:
```xml
<select id="selectByCondition" parameterType="map" resultType="com.example.entity.User">
SELECT * FROM user WHERE
<if test="name != null and name != ''">
name = #{name}
</if>
<if test="age != null">
AND age = #{age}
</if>
<if test="gender != null and gender != ''">
AND gender = #{gender}
</if>
</select>
```
这个查询语句可以根据传入的 Map 参数中的 name、age、gender 字段进行条件查询。其中,`#{}` 表示参数占位符,MyBatis 会自动将 Map 中对应的值填充进去。
最后,我们可以在 Service 中调用 Mapper 的查询方法,例如:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> selectByCondition(String name, Integer age, String gender) {
Map<String, Object> condition = new HashMap<>();
condition.put("name", name);
condition.put("age", age);
condition.put("gender", gender);
return userMapper.selectByCondition(condition);
}
}
```
这个 Service 方法会将传入的查询条件封装成 Map,然后调用 Mapper 的查询方法进行查询,并返回结果列表。
相关推荐
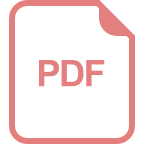














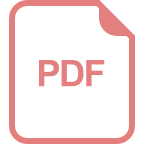