springboot mybatis 修改表A或表B或表C中的一个编码并且是唯一 的接口怎么写
时间: 2024-03-27 17:39:32 浏览: 17
首先,你需要在Spring Boot项目中集成MyBatis框架,然后定义一个Mapper接口,该接口中应该定义一个方法来执行修改操作。假设你要修改的表是表A,表中有一个名为code的唯一编码字段,方法名为updateCode:
```
@Mapper
public interface TableAMapper {
@Update("UPDATE table_a SET code=#{newCode} WHERE code=#{oldCode}")
int updateCode(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
}
```
这里使用了MyBatis的注解@Mapper和@Update,其中@Mapper指示该接口是一个Mapper,@Update指示该方法执行一个更新操作。方法的参数中使用了@Param注解,该注解用于指定方法参数对应的SQL语句中的参数名称。
在该方法中,我们执行了一条UPDATE语句,将表A中code字段值为oldCode的记录的code字段值修改为newCode。注意,这里使用了MyBatis的#{}语法,该语法可以将参数值安全地插入到SQL语句中,避免了SQL注入的风险。
如果你需要修改表B或表C中的唯一编码字段,只需要在对应的Mapper接口中定义类似的方法即可。
相关问题
springboot mybatis 在表A表B表C中修改编码并且是唯一 的接口怎么写
要在多表中修改编码并确保唯一性,需要进行一些复杂的逻辑处理。下面是一个示例实现。
假设有三张表:A、B、C,它们的编码字段分别为 ACode、BCode、CCode,并且都是唯一的。现在需要修改 A 表的 ACode,并且要确保修改后的 ACode 在 B 表和 C 表中都是唯一的。
首先,需要在 Mapper 文件中编写修改 A 表的语句,例如:
```xml
<update id="updateACode" parameterType="map">
UPDATE A SET ACode = #{newCode} WHERE ACode = #{oldCode}
</update>
```
这个语句会将 A 表中 ACode 等于 oldCode 的记录的 ACode 修改为 newCode。
接下来,需要编写查询 B 表和 C 表的语句,以检查新的 ACode 在这两张表中是否唯一。例如:
```xml
<select id="countBCode" parameterType="string" resultType="int">
SELECT COUNT(*) FROM B WHERE BCode = #{newCode}
</select>
<select id="countCCode" parameterType="string" resultType="int">
SELECT COUNT(*) FROM C WHERE CCode = #{newCode}
</select>
```
这两个查询语句会分别返回 B 表和 C 表中 BCode 或 CCode 等于 newCode 的记录数。
最后,需要在 Service 中编写一个事务方法,将上面的语句组合起来,实现修改 A 表的 ACode 并确保唯一性的逻辑。例如:
```java
@Service
public class MyService {
@Autowired
private AMapper aMapper;
@Autowired
private BMapper bMapper;
@Autowired
private CMapper cMapper;
@Transactional
public void updateACode(String oldCode, String newCode) {
// 修改 A 表的 ACode
Map<String, String> params = new HashMap<>();
params.put("oldCode", oldCode);
params.put("newCode", newCode);
aMapper.updateACode(params);
// 检查新的 ACode 在 B 表和 C 表中是否唯一
int countBCode = bMapper.countBCode(newCode);
int countCCode = cMapper.countCCode(newCode);
if (countBCode > 1 || countCCode > 1) {
// 如果不唯一,则抛出异常回滚事务
throw new RuntimeException("ACode is not unique");
}
}
}
```
这个方法会在事务中执行修改 A 表和检查唯一性的操作,如果新的 ACode 不唯一,则抛出异常回滚事务。
springboot mybatis 在表A表B表C中修改查询条件查出来的编码并且是唯一 的接口怎么写
首先,在 Spring Boot 中使用 MyBatis,需要在 pom.xml 中引入相应的依赖:
```xml
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
```
其次,需要在 application.properties 文件中配置 MyBatis 的相关参数,如数据源、Mapper 文件的位置等:
```properties
# 数据源配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# MyBatis 配置
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.type-aliases-package=com.example.entity
```
其中,`mybatis.mapper-locations` 指定了 Mapper 文件的位置,`mybatis.type-aliases-package` 指定了实体类的包名。
接下来,我们可以在 Mapper 文件中编写查询语句,例如:
```xml
<select id="selectByCondition" parameterType="map" resultType="com.example.entity.User">
SELECT * FROM user WHERE
<if test="name != null and name != ''">
name = #{name}
</if>
<if test="age != null">
AND age = #{age}
</if>
<if test="gender != null and gender != ''">
AND gender = #{gender}
</if>
</select>
```
这个查询语句可以根据传入的 Map 参数中的 name、age、gender 字段进行条件查询。其中,`#{}` 表示参数占位符,MyBatis 会自动将 Map 中对应的值填充进去。
最后,我们可以在 Service 中调用 Mapper 的查询方法,例如:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> selectByCondition(String name, Integer age, String gender) {
Map<String, Object> condition = new HashMap<>();
condition.put("name", name);
condition.put("age", age);
condition.put("gender", gender);
return userMapper.selectByCondition(condition);
}
}
```
这个 Service 方法会将传入的查询条件封装成 Map,然后调用 Mapper 的查询方法进行查询,并返回结果列表。
相关推荐
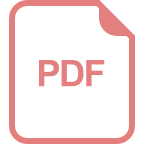











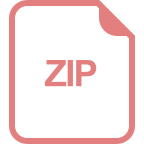