springboot mybatis 修改多表中的编码,修改后的编码不重复 接口怎么写
时间: 2024-03-27 17:40:52 浏览: 59
以下是一个示例的代码实现,可以根据实际情况进行修改:
```java
@Service
@Transactional
public class CodeService {
@Autowired
private SqlSessionTemplate sqlSessionTemplate;
public void updateCode(String oldCode, String newCode) {
// 1.查询需要修改的记录
List<Code> codeList = sqlSessionTemplate.selectList("CodeMapper.selectByCode", oldCode);
if (codeList == null || codeList.isEmpty()) {
throw new RuntimeException("找不到需要修改的记录");
}
// 2.修改记录
for (Code code : codeList) {
code.setCode(newCode);
}
// 3.检查新的编码是否已经存在
int count = sqlSessionTemplate.selectOne("CodeMapper.countByCode", newCode);
if (count > 0) {
throw new RuntimeException("新的编码已经存在");
}
// 4.更新编码字段
for (Code code : codeList) {
sqlSessionTemplate.update("CodeMapper.updateCode", code);
}
}
}
```
在上面的示例代码中,我们使用了 MyBatis 的 SqlSessionTemplate 来执行 SQL 语句,同时使用了 Spring 的事务注解来管理事务。具体的 SQL 语句可以根据实际情况进行修改。
以下是一个示例的接口代码,可以根据实际情况进行修改:
```java
@RestController
@RequestMapping("/api")
public class CodeController {
@Autowired
private CodeService codeService;
@PostMapping("/updateCode")
public Result updateCode(@RequestParam("oldCode") String oldCode,
@RequestParam("newCode") String newCode) {
try {
codeService.updateCode(oldCode, newCode);
return Result.success();
} catch (Exception e) {
return Result.error("修改编码失败:" + e.getMessage());
}
}
}
```
在上面的示例代码中,我们定义了一个 POST 请求的接口,接收两个参数 oldCode 和 newCode,分别表示需要修改的编码和修改后的编码。在接口的实现中,我们调用了 CodeService 类的 updateCode 方法来执行实际的修改操作,并根据操作结果返回不同的响应。需要注意的是,如果修改失败,我们需要返回错误信息,以便前端进行提示。
阅读全文
相关推荐
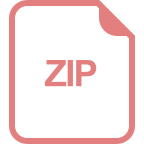


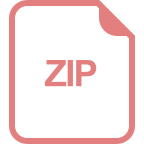
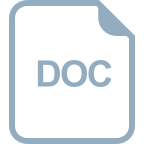
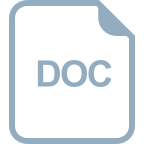
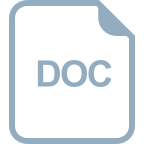
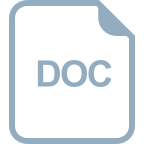
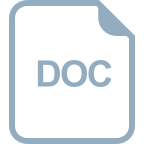
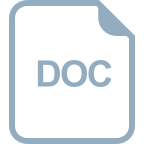
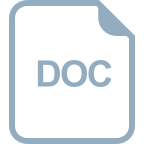
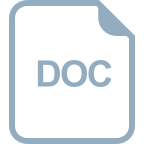
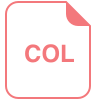
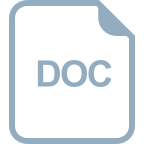
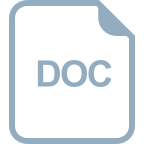
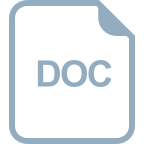

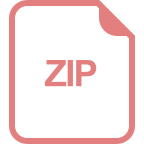