springboot mybatis 修改多表中的一个编码,先判断编码在哪个表中存在,再判断修改后的编码是否在多表编码字段中重复
时间: 2024-03-27 21:39:35 浏览: 50
要实现这个功能,可以在MyBatis的Mapper接口中定义一个方法,该方法需要执行以下操作:
1. 判断编码在哪个表中存在,可以使用SELECT语句查询各个表中是否存在该编码;
2. 判断修改后的编码是否在多表编码字段中重复,可以使用SELECT语句查询多个表中是否存在该编码;
3. 如果编码不存在或者修改后的编码不重复,则执行UPDATE语句修改编码;
下面是一个示例代码,假设要修改的编码为newCode,原编码为oldCode,要修改的表为table_a,table_b和table_c,编码字段名为code:
```
@Mapper
public interface CodeMapper {
@Select("SELECT COUNT(*) FROM table_a WHERE code=#{code}")
int checkTableA(@Param("code") String code);
@Select("SELECT COUNT(*) FROM table_b WHERE code=#{code}")
int checkTableB(@Param("code") String code);
@Select("SELECT COUNT(*) FROM table_c WHERE code=#{code}")
int checkTableC(@Param("code") String code);
@Select("SELECT COUNT(*) FROM (SELECT code FROM table_a UNION ALL SELECT code FROM table_b UNION ALL SELECT code FROM table_c) t WHERE code=#{code}")
int checkDuplicateCode(@Param("code") String code);
@Update("UPDATE table_a SET code=#{newCode} WHERE code=#{oldCode}")
int updateTableA(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
@Update("UPDATE table_b SET code=#{newCode} WHERE code=#{oldCode}")
int updateTableB(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
@Update("UPDATE table_c SET code=#{newCode} WHERE code=#{oldCode}")
int updateTableC(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
default boolean updateCode(String oldCode, String newCode) {
// 检查编码在哪个表中存在
boolean codeExistsInTableA = checkTableA(oldCode) > 0;
boolean codeExistsInTableB = checkTableB(oldCode) > 0;
boolean codeExistsInTableC = checkTableC(oldCode) > 0;
// 如果编码在多个表中都不存在,则无法修改
if (!codeExistsInTableA && !codeExistsInTableB && !codeExistsInTableC) {
return false;
}
// 检查修改后的编码是否重复
boolean newCodeExists = checkDuplicateCode(newCode) > 0;
if (newCodeExists) {
return false;
}
// 执行更新操作
if (codeExistsInTableA) {
updateTableA(oldCode, newCode);
}
if (codeExistsInTableB) {
updateTableB(oldCode, newCode);
}
if (codeExistsInTableC) {
updateTableC(oldCode, newCode);
}
return true;
}
}
```
在该代码中,我们定义了多个方法来执行各个操作,其中checkTableA、checkTableB和checkTableC方法用于检查编码是否在各个表中存在,checkDuplicateCode方法用于检查修改后的编码是否在多个表中重复,updateTableA、updateTableB和updateTableC方法用于执行修改操作。
最终我们在CodeMapper接口中定义了一个默认方法updateCode,该方法需要执行以上三个步骤,并返回修改结果的布尔值。
阅读全文
相关推荐
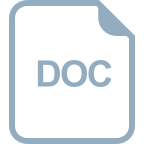
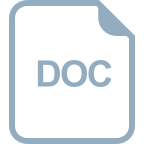
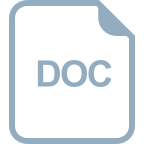









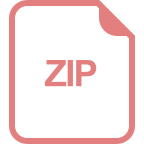


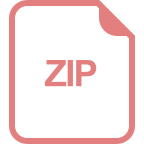

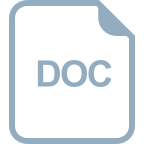
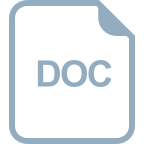
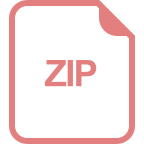