springboot mybatis 更改多表中的编码,更改后的编码在多表中是唯一的接口怎么写
时间: 2024-03-27 18:41:01 浏览: 79
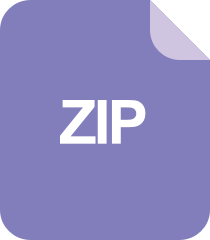
springboot+mybatis配置多数据源,通过注解自动切换
假设我们有两张表Product和Order,需要将Product表中编码为oldCode的商品编码更改为newCode,并且更改后的编码在Product表和Order表中都是唯一的。接口可以采用以下方式来实现:
```
@Mapper
public interface ProductMapper {
//查询编码为oldCode的商品信息
@Select("SELECT * FROM product WHERE code = #{oldCode}")
Product findProductByCode(String oldCode);
//更新编码为oldCode的商品编码为newCode
@Update("UPDATE product SET code = #{newCode} WHERE code = #{oldCode}")
void updateProductCode(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
//查询编码为newCode的商品数量
@Select("SELECT COUNT(*) FROM product WHERE code = #{newCode}")
int countProductByCode(String newCode);
//查询编码为newCode的订单数量
@Select("SELECT COUNT(*) FROM order WHERE product_code = #{newCode}")
int countOrderByProductCode(String newCode);
}
@Service
public class ProductService {
@Autowired
private ProductMapper productMapper;
@Transactional
public void updateProductCode(String oldCode, String newCode) throws Exception {
//查询编码为oldCode的商品信息
Product product = productMapper.findProductByCode(oldCode);
if (product == null) {
throw new Exception("商品编码为" + oldCode + "的商品不存在!");
}
//查询编码为newCode的商品数量
int countProduct = productMapper.countProductByCode(newCode);
if (countProduct > 0) {
throw new Exception("编码为" + newCode + "的商品已存在!");
}
//查询编码为newCode的订单数量
int countOrder = productMapper.countOrderByProductCode(newCode);
if (countOrder > 0) {
throw new Exception("编码为" + newCode + "的商品已存在订单中,不能更改!");
}
//更新编码为oldCode的商品编码为newCode
productMapper.updateProductCode(oldCode, newCode);
}
}
```
该接口实现了查询编码为oldCode的商品信息,更新编码为oldCode的商品编码为newCode,查询编码为newCode的商品数量,查询编码为newCode的订单数量,如果编码为newCode的商品已存在或者编码为newCode的商品已存在订单中则抛出异常。使用@Transactional注解将方法置为事务,保证在更新商品编码时,如果查询到编码为newCode的商品已存在或者编码为newCode的商品已存在订单中,则不会执行更新操作。
需要注意的是,这里只是一个简单的示例,实际的情况可能更加复杂,需要根据具体的业务需求来进行修改和完善。
阅读全文
相关推荐
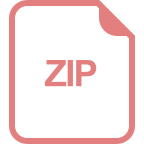
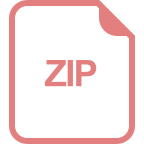








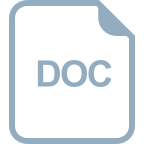
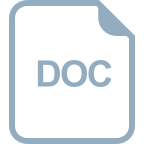
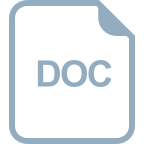


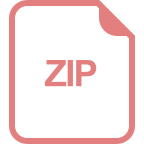
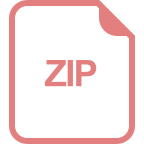