springboot mybatis 修改多表中的一个编码,修改后的编码在多表编码字段中不重复接口
时间: 2024-03-28 11:42:30 浏览: 25
你可以通过编写一个事务来实现修改多表中的一个编码的操作,并且保证修改后的编码在多表编码字段中不重复。
具体步骤如下:
1. 首先,在你的 DAO 层中定义一个方法,用于更新需要修改编码的表中的编码字段。例如,如果你需要修改的表是 `table1`,那么该方法可以如下所示:
```java
@Mapper
public interface Table1Mapper {
int updateCode(@Param("oldCode") String oldCode, @Param("newCode") String newCode);
}
```
其中 `oldCode` 是需要修改的编码,`newCode` 是修改后的编码。
2. 然后,在你的 Service 层中编写一个事务来保证多表修改的原子性。例如,如果你需要同时修改 `table1` 和 `table2` 两张表中的编码字段,那么该事务可以如下所示:
```java
@Service
public class MyService {
@Autowired
private Table1Mapper table1Mapper;
@Autowired
private Table2Mapper table2Mapper;
@Transactional
public void updateCode(String oldCode, String newCode) {
// 先更新 table1 中的编码字段
int result1 = table1Mapper.updateCode(oldCode, newCode);
if (result1 != 1) {
throw new RuntimeException("更新 table1 失败");
}
// 再更新 table2 中的编码字段
int result2 = table2Mapper.updateCode(oldCode, newCode);
if (result2 != 1) {
throw new RuntimeException("更新 table2 失败");
}
}
}
```
在上述代码中,我们使用 `@Transactional` 注解来表示该方法是一个事务,当其中任何一个更新操作失败时,都会将整个事务回滚。
3. 最后,在你的 Controller 层中调用该 Service 方法即可完成编码的修改。例如:
```java
@RestController
public class MyController {
@Autowired
private MyService myService;
@PostMapping("/updateCode")
public void updateCode(@RequestParam("oldCode") String oldCode, @RequestParam("newCode") String newCode) {
myService.updateCode(oldCode, newCode);
}
}
```
在上述代码中,我们使用 `@PostMapping` 注解来表示该方法处理 POST 请求,并使用 `@RequestParam` 注解来获取请求参数。
相关推荐
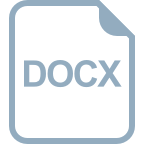
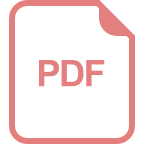
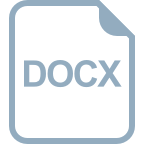
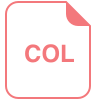
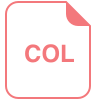
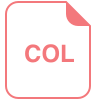
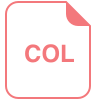
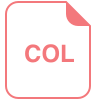









