c++在不使用filesystem库情况下实现递归复制文件目录
时间: 2024-09-12 08:16:35 浏览: 37
在C++中,不使用标准库`<filesystem>`的情况下实现递归复制文件目录可以手动操作`<fstream>`、`<dirent>`和`<sys/stat>`等头文件提供的功能。这里是一个简单的示例,展示了如何遍历目录并逐个复制文件:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <dirent.h>
#include <sys/stat.h>
// 检查是否为目录
bool is_directory(const std::string& path) {
struct stat sb;
return stat(path.c_str(), &sb) == 0 && S_ISDIR(sb.st_mode);
}
// 复制单个文件
void copy_file(const std::string& src, const std::string& dst) {
std::ifstream in(src, std::ios::binary);
if (!in) {
throw std::runtime_error("Failed to open source file");
}
std::ofstream out(dst, std::ios::binary);
if (!out) {
throw std::runtime_error("Failed to create destination file");
}
out << in.rdbuf();
in.close();
out.close();
}
// 递归复制目录
void recursive_copy(const std::string& src_path, const std::string& dst_path) {
if (is_directory(src_path)) {
mkdir(dst_path, 0755); // 创建目录
DIR* dir = opendir(src_path.c_str());
if (!dir) {
perror("opendir");
return;
}
dirent* entry;
while ((entry = readdir(dir))) {
std::string entry_name = entry->d_name;
if (entry_name != "." && entry_name != "..") { // 避免当前目录和上一级目录
recursive_copy(src_path + "/" + entry_name, dst_path + "/" + entry_name);
}
}
closedir(dir);
} else {
copy_file(src_path, dst_path);
}
}
阅读全文
相关推荐
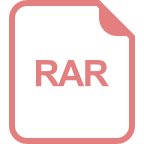
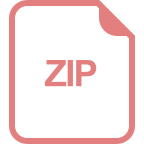
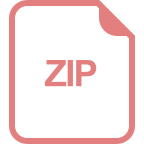

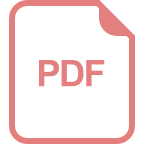
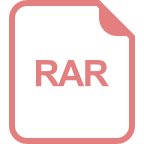
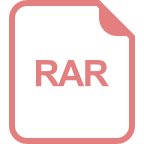
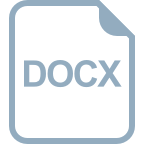
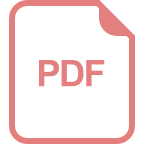
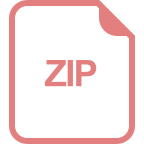
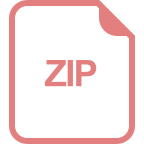
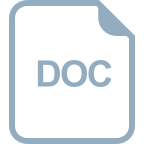
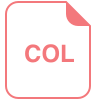





