c++实现复制文件夹及子目录文件
时间: 2024-09-12 09:16:23 浏览: 15
在C++中,复制文件夹及其所有子目录的内容通常涉及到遍历目录结构、创建目标路径以及逐个复制文件。这需要使用到操作系统API或者第三方库,比如Boost/filesystem。以下是一个简化的示例,展示了如何使用Boost库来复制整个目录:
```cpp
#include <boost/filesystem.hpp>
#include <iostream>
#include <algorithm>
// 检查源文件是否是一个目录
bool is_directory(const boost::filesystem::path& path) {
return boost::filesystem::is_directory(path);
}
// 复制文件
void copy_file(const boost::filesystem::path& src, const boost::filesystem::path& dst) {
if (!boost::filesystem::exists(src)) {
std::cerr << "Source file not found: " << src << std::endl;
return;
}
boost::filesystem::copy(src, dst);
}
// 复制目录递归
void copy_directory(const boost::filesystem::path& src, const boost::filesystem::path& dst) {
if (!is_directory(src)) {
std::cerr << "Expected a directory, but got: " << src << std::endl;
return;
}
for (const auto& entry : boost::filesystem::directory_iterator(src)) {
// 如果是目录,递归复制
if (is_directory(entry)) {
copy_directory(entry.path(), dst / entry.path().filename());
} else { // 是文件,则直接复制
copy_file(entry.path(), dst / entry.path().filename());
}
}
}
int main() {
try {
boost::filesystem::path src_dir = "/path/to/source/directory";
boost::filesystem::path dest_dir = "/path/to/destination/directory";
if (!boost::filesystem::exists(dest_dir)) {
boost::filesystem::create_directories(dest_dir); // 创建目标目录如果不存在
}
copy_directory(src_dir, dest_dir);
std::cout << "Directory copied successfully!" << std::endl;
} catch (const boost::filesystem::filesystem_error& e) {
std::cerr << "Error copying directory: " << e.what() << std::endl;
}
return 0;
}
```
相关推荐
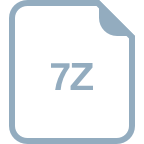
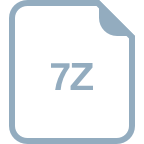
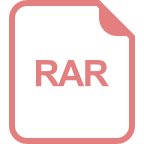














