编写一个程序,计算数组中元素的总和,直到总和达到或超过给定的最大值。如果总和超过数组结束前的最大值,请停止并打印总和。
时间: 2024-10-20 12:08:12 浏览: 0
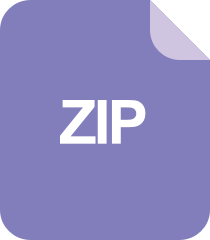
有一个int数组{1,3,5,-2,4,6},要求获取:最大值、最小值、元素和、平均值

要编写这个程序,你可以使用Java中的循环结构(如`for`或`while`)遍历数组,同时跟踪当前的总和。当总和达到或超过最大值时,就停止计算并打印结果。以下是一个简单的示例:
```java
import java.util.Scanner;
public class SumUntilMax {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 提示用户输入数组长度和最大值
System.out.print("Enter the length of the array: ");
int arrayLength = scanner.nextInt();
System.out.print("Enter the maximum sum to reach: ");
int maxSum = scanner.nextInt();
// 假设数组元素都是整数
int[] array = new int[arrayLength];
int currentSum = 0;
// 遍历数组
for (int i = 0; i < arrayLength && currentSum <= maxSum; i++) {
System.out.print("Enter element " + (i+1) + ": ");
array[i] = scanner.nextInt();
currentSum += array[i];
}
// 打印总和
if (currentSum <= maxSum) {
System.out.println("Sum reached or exceeded the limit: " + currentSum);
} else {
System.out.println("Sum exceeded the limit before reaching it: " + currentSum);
}
scanner.close();
}
}
```
在这个程序中,我们首先获取用户的输入,然后用`for`循环遍历数组,每次从用户那里获取一个新元素并将之加到`currentSum`上。只要`currentSum`没有超过`maxSum`,我们就继续循环。一旦`currentSum`超过`maxSum`,就跳出循环并打印相应的消息。
运行这个程序后,你可以根据提示逐个输入数组元素,直到满足条件为止。
阅读全文
相关推荐
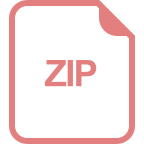
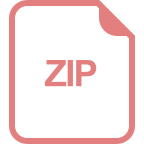
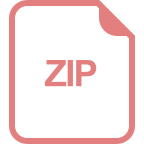
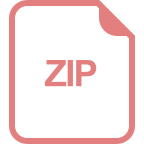
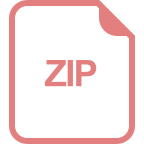
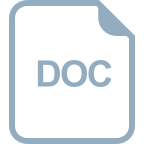
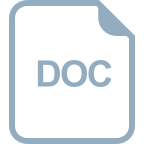
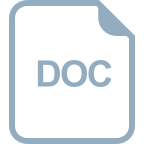







