代码生成(用python实现椭圆曲线的签名)
时间: 2023-07-12 11:33:56 浏览: 117
以下是一个用Python实现椭圆曲线的签名的简单示例代码。请注意,这只是一个示例代码,实际实现需要更复杂的算法和安全性考虑。
```python
import hashlib
import random
# 椭圆曲线的参数设置(选择一种曲线)
p = 2**256 - 2**32 - 977
a = 0
b = 7
Gx = 55066263022277343669578718895168534326250603453777594175500187360389116729240
Gy = 32670510020758816978083085130507043184471273380659243275938904335757337482424
n = 115792089237316195423570985008687907852837564279074904382605163141518161494337
# 定义椭圆曲线点类
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return f"({self.x}, {self.y})"
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def __add__(self, other):
if self == other:
return self.double()
elif self.x is None:
return other
elif other.x is None:
return self
elif self.x == other.x:
return Point(None, None)
else:
slope = (other.y - self.y) // (other.x - self.x)
x3 = slope ** 2 - self.x - other.x
y3 = slope * (self.x - x3) - self.y
return Point(x3, y3)
def double(self):
if self.y == 0:
return Point(None, None)
else:
slope = (3 * self.x ** 2) // (2 * self.y)
x3 = slope ** 2 - 2 * self.x
y3 = slope * (self.x - x3) - self.y
return Point(x3, y3)
def __mul__(self, other):
if other == 0:
return Point(None, None)
elif other == 1:
return self
else:
Q = self.double()
if other % 2 == 0:
return Q * (other // 2)
else:
return self + Q * (other // 2)
# 定义哈希函数(使用SHA-1)
def sha1(message):
return int(hashlib.sha1(message).hexdigest(), 16)
# 定义签名函数
def sign(private_key, message):
z = sha1(message.encode())
k = random.randint(0, n-1)
r = (k * Point(Gx, Gy)).x % n
s = (pow(k, n-2, n) * (z + r * private_key)) % n
return (r, s)
# 定义验证签名函数
def verify(public_key, message, signature):
z = sha1(message.encode())
r, s = signature
w = pow(s, n-2, n)
u1 = (z * w) % n
u2 = (r * w) % n
P = u1 * Point(Gx, Gy) + u2 * public_key
return r == (P.x % n)
# 生成公私钥对
private_key = random.randint(1, n-1)
public_key = private_key * Point(Gx, Gy)
# 对消息进行签名并验证
message = "Hello, world!"
signature = sign(private_key, message)
print("Signature:", signature)
print("Verify:", verify(public_key, message, signature))
```
请注意,此示例代码中使用的是一条256位的椭圆曲线,密钥长度为256比特。在实际实现中,应该根据具体需求选择合适的曲线和密钥长度,并对代码进行更多的安全性考虑。
相关推荐
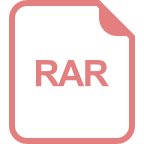
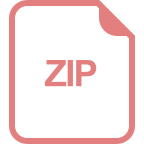














