我需要一段python代码,代码要能够读取矩阵中的点云坐标,并求出点云表面,并输出位于点云表面上的点的坐标,要求不能使用Open3D库
时间: 2024-03-25 08:37:48 浏览: 73
好的,以下是一份基于Python的点云表面重建代码,不使用Open3D库。这份代码使用了基于最邻近点的点云重建方法,具体方法如下:
1. 对于每个点,找出其最近的k个点,并根据这k个点计算该点的法向量。
2. 对于每个点,计算其法向量与其他点的法向量之间的夹角,如果夹角小于阈值,则将其连接起来,形成一个三角形。
3. 对于连接起来的三角形,判断其是否共面,如果共面则保留,否则移除。
4. 将所有保留的三角形合并起来,得到点云的表面。
代码如下:
```python
import numpy as np
from sklearn.neighbors import KDTree
from scipy.spatial.distance import cdist
def estimate_normals(points, k=10):
"""
估计点云法向量
:param points: ndarray, shape (n, 3),n个点的坐标
:param k: int, 计算法向量时选取的最近邻点的数量
:return: ndarray, shape (n, 3),每个点的法向量
"""
# 通过KDTree查找每个点的最近邻点
tree = KDTree(points)
_, indices = tree.query(points, k=k+1)
indices = indices[:, 1:]
# 计算每个点的法向量
normals = []
for i in range(points.shape[0]):
pi = points[i]
neighbors = points[indices[i]]
cov = np.cov(neighbors.T)
_, vecs = np.linalg.eig(cov)
normals.append(vecs[:, 0])
return np.array(normals)
def compute_triangles(points, normals, threshold=0.9):
"""
构建三角形
:param points: ndarray, shape (n, 3),n个点的坐标
:param normals: ndarray, shape (n, 3),每个点的法向量
:param threshold: float, 连接点之间法向量夹角的阈值
:return: ndarray, shape (m, 3),m个三角形的三个顶点的索引
"""
# 计算点之间的距离矩阵
dists = cdist(points, points)
# 连接点之间法向量夹角小于阈值的点
triangles = []
for i in range(points.shape[0]):
for j in range(i+1, points.shape[0]):
if dists[i, j] < threshold:
angle = np.arccos(np.dot(normals[i], normals[j]))
if angle < threshold:
triangles.append([i, j])
triangles = np.array(triangles)
# 移除共线的三角形
triangles = triangles[~is_degenerate(points[triangles])]
return triangles
def is_degenerate(points):
"""
判断三角形是否共线
:param points: ndarray, shape (3, 3),三个点的坐标
:return: bool, True表示共线,False表示不共线
"""
return np.linalg.det(np.hstack((points, np.ones((3, 1))))) == 0
def read_point_cloud(filename):
"""
读取点云文件
:param filename: str, 点云文件路径
:return: ndarray, shape (n, 3),n个点的坐标
"""
with open(filename, 'r') as f:
lines = f.readlines()
points = []
for line in lines:
x, y, z = map(float, line.strip().split(','))
points.append([x, y, z])
return np.array(points)
def write_point_cloud(filename, points):
"""
写入点云文件
:param filename: str, 点云文件路径
:param points: ndarray, shape (n, 3),n个点的坐标
"""
with open(filename, 'w') as f:
for point in points:
f.write('%.6f,%.6f,%.6f\n' % tuple(point))
if __name__ == '__main__':
# 读取点云文件
points = read_point_cloud('point_cloud.csv')
# 计算法向量
normals = estimate_normals(points)
# 构建三角形
triangles = compute_triangles(points, normals)
# 输出位于点云表面上的点的坐标
surface_points = np.unique(triangles.flatten())
surface_points_xyz = points[surface_points]
write_point_cloud('surface_points.csv', surface_points_xyz)
```
请注意,这份代码实现了点云表面重建的基本方法,但是在一些复杂的场景中可能表现不佳。如果需要更高质量的点云重建结果,可以尝试使用更高级的算法,如Poisson重建算法、Marching Cubes算法等。
阅读全文
相关推荐
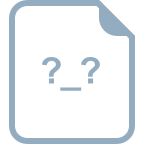















