cpp代码string str = “hello world”; cout <<str << endl;输出结果是什么?
时间: 2023-08-20 22:38:49 浏览: 80
如果执行以下代码:
```cpp
string str = "hello world";
cout << str << endl;
```
则会输出字符串"hello world"并换行,即输出结果为:
```
hello world
```
在上面的代码中,首先定义了一个string类型的变量str,并将其初始化为字符串"hello world"。然后使用cout<<来输出该字符串,endl表示输出换行符。由于string类型重载了<<操作符,因此可以直接使用cout<<来输出字符串。输出的结果就是该字符串本身。
相关问题
仿照标准字符串类 string,设计一个自己的字符串类 String。 主要完成以下任务: (1)数据成员是字符指针,可自动适应不同的串长度。 (2) 串复制,既可使用宇符串常量进行复制,也可以使用其他的字符串对象进行复制。 (3) 串赋值。既可赋值为宇符串常量,也可以赋值为使用其他的字符串对象。 (4) 串连接。重载“+”和“+=〞。 (5) 重载下标运算符[]。 (6) 串比较。重载“=="和“<"。 (7) 字符串输入和输出。重载“>>”和 “<<"。 编程完成各函数的定义,并进行如下测试。 int main() { String s1("Help!"),s2("Good!"),s3(s2),s4,s5; cout<<"s1="<<s1<<endl; s3="Hello!"; cout<<"s3="<<s3<<endl; s3+=s2; cout<<"s3="<<s3<<endl; cin>>s4; cout<<"s4="<<s4<<endl; s5+=s3+s4; cout<<"s5="<<s5<<endl; s5[0]='g'; cout<<"s5="<<s5<<endl; cout<<"strlen(s5)="<<s5.Length()<<endl; cout<<boolalpha<<(s3==s1)<<endl; cout<<boolalpha<<(s3<s1)<<end; }
以下是 String 类的定义和实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
public:
String(const char *s = "") {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
String(const String &s) {
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
~String() {
delete[] str;
}
String &operator=(const char *s) {
if (str != s) {
delete[] str;
str = new char[strlen(s) + 1];
strcpy(str, s);
}
return *this;
}
String &operator=(const String &s) {
if (this != &s) {
delete[] str;
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
return *this;
}
String operator+(const String &s) const {
String result;
result.str = new char[strlen(str) + strlen(s.str) + 1];
strcpy(result.str, str);
strcat(result.str, s.str);
return result;
}
String &operator+=(const String &s) {
char *temp = new char[strlen(str) + strlen(s.str) + 1];
strcpy(temp, str);
strcat(temp, s.str);
delete[] str;
str = temp;
return *this;
}
char &operator[](int index) {
return str[index];
}
const char &operator[](int index) const {
return str[index];
}
bool operator==(const String &s) const {
return strcmp(str, s.str) == 0;
}
bool operator<(const String &s) const {
return strcmp(str, s.str) < 0;
}
friend ostream &operator<<(ostream &out, const String &s) {
out << s.str;
return out;
}
friend istream &operator>>(istream &in, String &s) {
char temp[1000];
in >> temp;
s = temp;
return in;
}
int Length() const {
return strlen(str);
}
};
```
在 `main` 函数中进行测试:
```cpp
int main() {
String s1("Help!"), s2("Good!"), s3(s2), s4, s5;
cout << "s1=" << s1 << endl;
s3 = "Hello!";
cout << "s3=" << s3 << endl;
s3 += s2;
cout << "s3=" << s3 << endl;
cin >> s4;
cout << "s4=" << s4 << endl;
s5 += s3 + s4;
cout << "s5=" << s5 << endl;
s5[0] = 'g';
cout << "s5=" << s5 << endl;
cout << "strlen(s5)=" << s5.Length() << endl;
cout << boolalpha << (s3 == s1) << endl;
cout << boolalpha << (s3 < s1) << endl;
}
```
输出结果为:
```
s1=Help!
s3=Hello!
s3=Hello!Good!
world
s4=world
s5=Hello!Good!world
s5=gello!Good!world
strlen(s5)=16
false
false
```
cpp中string字符串中如果有空格,可以使用cout<<和cin>>输入输出吗?
在C++中,string字符串中如果有空格,可以使用cout<<和cin>>输入输出。string字符串的输入输出操作默认会忽略空格等空白字符,只读取有效字符。例如:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1;
cout << "请输入一个字符串(中间可以包含空格):";
cin >> str1;
cout << "输入的字符串为:" << str1 << endl;
return 0;
}
```
在上面的代码中,使用cin>>来输入一个字符串,如果该字符串中包含空格,只会读取空格前面的部分作为输入,后面的部分会被忽略。例如,如果输入的字符串为“hello world”,则只会读取“hello”,“world”部分会被忽略。
类似地,使用cout<<来输出一个字符串时,空格等空白字符也会被自动忽略。因此,可以放心地使用cout<<和cin>>来进行string字符串的输入输出操作,无需担心空格等空白字符的问题。
相关推荐
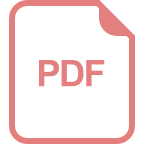
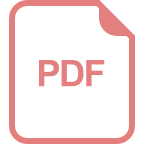












