C++定义一个字符串类,重载的运算符包括:=、+、+=、>、<、>=、<=、==、!=。其中,除=、+=运算符用成员函数定义外、其余的运算符要求用友元方式定义。
时间: 2024-10-20 21:18:15 浏览: 64
在C++中,定义一个自定义字符串类(通常称为`std::string`,但我们这里假设创建一个新的名为`MyString`的类),并重载一些基本的运算符,可以按照以下步骤进行:
首先,定义`MyString`类,并包含必要的头文件:
```cpp
#include <iostream>
#include <string>
class MyString {
private:
std::string str;
public:
// 构造函数和其他成员函数
MyString(const char* str = "");
MyString& operator=(const MyString& other); // 等号赋值
MyString operator+(const MyString& other) const; // 连接运算符
friend bool operator>(const MyString& a, const MyString& b); // 比较运算符大于
friend bool operator<(const MyString& a, const MyString& b); // 小于
friend bool operator>=(const MyString& a, const MyString& b); // 大于等于
friend bool operator<=(const MyString& a, const MyString& b); // 小于等于
friend bool operator==(const MyString& a, const MyString& b); // 等于
friend bool operator!=(const MyString& a, const MyString& b); // 不等于
};
```
然后,实现每个运算符的对应功能:
- `operator=`(赋值):将其他字符串的内容复制到当前实例中。
- `operator+`(连接):将两个字符串拼接到一起形成一个新的字符串。
友元函数部分,因为它们直接访问类的私有数据,所以需要声明为友元:
```cpp
bool operator>(const MyString& a, const MyString& b) {
return a.str > b.str;
}
// 其他友元函数的实现类似
```
注意:`+=`和`=`同样可以用成员函数实现,因为它们可以直接修改对象的状态。
最后,你可以像操作普通字符串一样使用这些重载的运算符:
```cpp
int main() {
MyString s1("Hello");
MyString s2("World");
// 使用重载的运算符进行比较
if (s1 > s2) {
std::cout << "s1 is greater than s2" << std::endl;
}
// 使用+运算符连接字符串
MyString combined = s1 + " " + s2;
std::cout << "Combined string: " << combined << std::endl;
return 0;
}
```
阅读全文
相关推荐
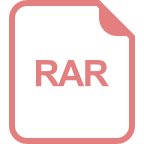
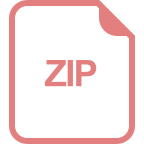
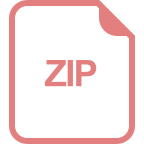















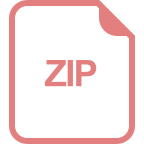