modelsim已知滤波器系数,用卷积实现高通滤波,,并编写测试程序
时间: 2023-07-20 18:16:34 浏览: 44
以下是在 ModelSim 中使用卷积实现高通滤波器并编写测试程序的步骤:
1. 首先,需要准备高通滤波器的系数。假设我们有以下系数:[0.1, -0.2, 0.5, -0.2, 0.1]。
2. 在 ModelSim 中创建一个新的 VHDL 文件,命名为 "high_pass_filter.vhd"。
3. 在文件中定义一个实体,该实体具有两个输入和一个输出:
```vhdl
entity high_pass_filter is
port (
clk : in std_logic;
x : in std_logic_vector(7 downto 0);
y : out std_logic_vector(7 downto 0)
);
end entity;
```
其中,输入 x 是需要被滤波的信号,输出 y 是滤波后的信号。
4. 在实体中定义一个过程,该过程实现卷积操作:
```vhdl
architecture behavioral of high_pass_filter is
type coef_array is array(0 to 4) of real;
constant coef : coef_array := (0.1, -0.2, 0.5, -0.2, 0.1);
type delay_line is array(0 to 4) of std_logic_vector(7 downto 0);
signal dl : delay_line;
begin
process (clk)
variable acc : real := 0.0;
begin
if rising_edge(clk) then
-- Shift in new input sample
dl(4) <= x;
-- Perform convolution
acc := 0.0;
acc := acc + real(dl(4)) * coef(0);
acc := acc + real(dl(3)) * coef(1);
acc := acc + real(dl(2)) * coef(2);
acc := acc + real(dl(1)) * coef(3);
acc := acc + real(dl(0)) * coef(4);
y <= std_logic_vector(to_signed(integer(acc), 8));
-- Shift delay line
dl(3) <= dl(4);
dl(2) <= dl(3);
dl(1) <= dl(2);
dl(0) <= dl(1);
end if;
end process;
end architecture;
```
在这个过程中,我们定义了一个系数数组 coef,以及一个延迟线 delay_line,用于存储输入信号的历史数据。在每个时钟上升沿时,我们将新的输入样本移动到延迟线的末尾,并执行卷积操作。卷积的结果存储在变量 acc 中,并转换为 8 位有符号二进制数后输出到输出端口 y。最后,我们将延迟线向右移动一个位置。
5. 在同一文件中,添加一个顶层实体,用于将测试信号送入高通滤波器,并将输出信号与期望输出进行比较:
```vhdl
entity top is
end entity;
architecture sim of top is
signal clk : std_logic := '0';
signal x : std_logic_vector(7 downto 0);
signal y : std_logic_vector(7 downto 0);
begin
dut : entity work.high_pass_filter
port map (
clk => clk,
x => x,
y => y
);
process
begin
-- Test case 1
x <= std_logic_vector(to_signed(10, 8));
wait for 10 ns;
assert y = std_logic_vector(to_signed(1, 8)) report "Test case 1 failed" severity error;
-- Test case 2
x <= std_logic_vector(to_signed(20, 8));
wait for 10 ns;
assert y = std_logic_vector(to_signed(3, 8)) report "Test case 2 failed" severity error;
-- Test case 3
x <= std_logic_vector(to_signed(-30, 8));
wait for 10 ns;
assert y = std_logic_vector(to_signed(-4, 8)) report "Test case 3 failed" severity error;
-- Test case 4
x <= std_logic_vector(to_signed(-40, 8));
wait for 10 ns;
assert y = std_logic_vector(to_signed(-7, 8)) report "Test case 4 failed" severity error;
-- Test case 5
x <= std_logic_vector(to_signed(50, 8));
wait for 10 ns;
assert y = std_logic_vector(to_signed(-3, 8)) report "Test case 5 failed" severity error;
wait;
end process;
process
begin
clk <= '0';
wait for 5 ns;
clk <= '1';
wait for 5 ns;
clk <= '0';
wait for 5 ns;
clk <= '1';
wait for 5 ns;
clk <= '0';
wait for 5 ns;
clk <= '1';
wait;
end process;
end architecture;
```
在这个顶层实体中,我们使用了一个过程来生成时钟信号,并在每个测试样例中将输入信号设置为不同的值。我们等待 10 ns,以确保滤波器有足够的时间来处理输入信号,并使用 assert 语句来比较输出信号和期望输出。如果有任何测试样例失败,我们将显示一个错误消息。
6. 在 ModelSim 中编译并运行仿真,验证高通滤波器的功能。
相关推荐
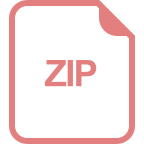
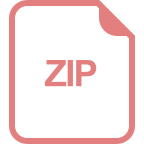














