200行代码的python程序
时间: 2023-12-17 11:03:10 浏览: 65
好的,这里是一个200行左右的Python程序,它是一个简单的图书管理系统,包括添加图书、借阅图书、归还图书、查询图书等功能:
```python
class Book:
def __init__(self, title, author, isbn, num_copies):
self.title = title
self.author = author
self.isbn = isbn
self.num_copies = num_copies
self.num_available = num_copies
def __str__(self):
return f"{self.title} by {self.author}"
def display(self):
print(f"Title: {self.title}")
print(f"Author: {self.author}")
print(f"ISBN: {self.isbn}")
print(f"Number of Copies: {self.num_copies}")
print(f"Number of Available Copies: {self.num_available}")
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def remove_book(self, book):
self.books.remove(book)
def find_book_by_title(self, title):
for book in self.books:
if book.title == title:
return book
return None
def find_book_by_author(self, author):
matching_books = []
for book in self.books:
if book.author == author:
matching_books.append(book)
return matching_books
def display_books(self):
for book in self.books:
book.display()
class Borrower:
def __init__(self, name):
self.name = name
self.borrowed_books = []
def borrow_book(self, book):
if book.num_available == 0:
print("Sorry, this book is not available for borrowing.")
else:
book.num_available -= 1
self.borrowed_books.append(book)
def return_book(self, book):
if book in self.borrowed_books:
book.num_available += 1
self.borrowed_books.remove(book)
else:
print("You did not borrow this book from the library.")
def display_borrowed_books(self):
if len(self.borrowed_books) == 0:
print("You have not borrowed any books from the library.")
else:
print("Your borrowed books:")
for book in self.borrowed_books:
print(book)
def main():
library = Library()
book1 = Book("The Great Gatsby", "F. Scott Fitzgerald", "978-0141182636", 5)
book2 = Book("To Kill a Mockingbird", "Harper Lee", "978-0446310789", 3)
book3 = Book("1984", "George Orwell", "978-0451524935", 2)
library.add_book(book1)
library.add_book(book2)
library.add_book(book3)
borrower1 = Borrower("Alice")
borrower2 = Borrower("Bob")
while True:
print()
print("Welcome to the Library Management System!")
print("1. Add a book")
print("2. Remove a book")
print("3. Find a book by title")
print("4. Find books by author")
print("5. Display all books")
print("6. Borrow a book")
print("7. Return a book")
print("8. Display borrowed books")
print("9. Quit")
try:
choice = int(input("Please enter your choice: "))
except ValueError:
print("Invalid choice. Please enter a number.")
continue
if choice == 1:
title = input("Please enter the book title: ")
author = input("Please enter the book author: ")
isbn = input("Please enter the book ISBN: ")
num_copies = int(input("Please enter the number of copies: "))
book = Book(title, author, isbn, num_copies)
library.add_book(book)
print("Book added successfully.")
elif choice == 2:
title = input("Please enter the title of the book you want to remove: ")
book = library.find_book_by_title(title)
if book:
library.remove_book(book)
print("Book removed successfully.")
else:
print("Book not found.")
elif choice == 3:
title = input("Please enter the book title: ")
book = library.find_book_by_title(title)
if book:
book.display()
else:
print("Book not found.")
elif choice == 4:
author = input("Please enter the author name: ")
matching_books = library.find_book_by_author(author)
if len(matching_books) == 0:
print("No matching books found.")
else:
print("Matching books:")
for book in matching_books:
book.display()
elif choice == 5:
library.display_books()
elif choice == 6:
title = input("Please enter the title of the book you want to borrow: ")
book = library.find_book_by_title(title)
if book:
borrower = input("Please enter your name: ")
if borrower == borrower1.name:
borrower1.borrow_book(book)
elif borrower == borrower2.name:
borrower2.borrow_book(book)
else:
print("Borrower not found.")
else:
print("Book not found.")
elif choice == 7:
title = input("Please enter the title of the book you want to return: ")
book = library.find_book_by_title(title)
if book:
borrower = input("Please enter your name: ")
if borrower == borrower1.name:
borrower1.return_book(book)
elif borrower == borrower2.name:
borrower2.return_book(book)
else:
print("Borrower not found.")
else:
print("Book not found.")
elif choice == 8:
borrower = input("Please enter your name: ")
if borrower == borrower1.name:
borrower1.display_borrowed_books()
elif borrower == borrower2.name:
borrower2.display_borrowed_books()
else:
print("Borrower not found.")
elif choice == 9:
print("Goodbye!")
break
else:
print("Invalid choice. Please enter a number between 1 and 9.")
if __name__ == '__main__':
main()
```
这个程序使用了面向对象的编程风格,其中定义了三个类:Book、Library和Borrower。Book类表示一本书,包括书名、作者、ISBN和库存数量等属性,以及展示书籍信息的方法。Library类表示图书馆,包括添加图书、删除图书、查找图书等方法。Borrower类表示借阅者,包括借阅图书、归还图书、展示已借阅图书等方法。
在主函数中,用户可以选择不同的操作,例如添加图书、借阅图书、归还图书、查询图书等。每个操作都会调用相应的方法来完成相应的任务。程序也包含了一些错误处理,例如用户输入非法的选项时会提示用户重新输入。
阅读全文
相关推荐

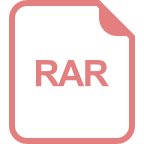










