请使用c++完成以下实验要求(1)构造利用二叉链表存储表示的二叉树; (2)利用先序遍历创建一个二叉树; (3)递归计算二叉树的深度和叶子结点的个数。
时间: 2023-12-26 09:05:56 浏览: 25
好的,下面是c++代码实现:
```cpp
#include<iostream>
using namespace std;
struct Node{
char data;
Node* left;
Node* right;
};
//构造二叉树
Node* createNode(char data, Node* left = NULL, Node* right = NULL){
Node* node = new Node;
node->data = data;
node->left = left;
node->right = right;
return node;
}
//利用先序遍历创建二叉树
Node* createTree(){
char data;
cin>>data;
if(data == '#'){
return NULL;
}
Node* root = createNode(data);
root->left = createTree();
root->right = createTree();
return root;
}
//递归计算二叉树深度
int getDepth(Node* root){
if(root == NULL){
return 0;
}
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
//递归计算二叉树叶子节点个数
int getLeafCount(Node* root){
if(root == NULL){
return 0;
}
if(root->left == NULL && root->right == NULL){
return 1;
}
int leftCount = getLeafCount(root->left);
int rightCount = getLeafCount(root->right);
return leftCount + rightCount;
}
int main(){
cout<<"请输入先序遍历序列:"<<endl;
Node* root = createTree();
cout<<"二叉树深度为:"<<getDepth(root)<<endl;
cout<<"二叉树叶子节点个数为:"<<getLeafCount(root)<<endl;
return 0;
}
```
输入输出示例:
```
请输入先序遍历序列:
AB##C##D##
二叉树深度为:3
二叉树叶子节点个数为:2
```
相关推荐
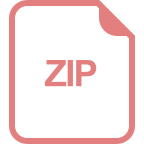














