文件in.txt(测试数据) 共1行,是一个算式算式包含字符: “(”、“)”、“0-9”、“+”、“-”、“*”、“/”、“^”。(“/”用整数除法,算式长度<=50,其中所有数据在2^31-1的范围内)。写一段程序读取这个文件中的算式并算出结果
时间: 2023-11-04 13:05:58 浏览: 37
以下是使用Python语言实现的计算代码:
```python
# 读取文件中的算式
with open('in.txt', 'r') as f:
expr = f.readline().strip()
# 定义计算函数
def calculate(expr):
# 将算式中的字符分离出来
tokens = []
num = ''
for ch in expr:
if ch.isdigit():
num += ch
else:
if num:
tokens.append(int(num))
num = ''
if ch in '+-*/^()':
tokens.append(ch)
if num:
tokens.append(int(num))
# 定义优先级
priority = {
'^': 3,
'*': 2,
'/': 2,
'+': 1,
'-': 1,
'(': 0
}
# 定义栈和输出队列
stack = []
output = []
# 中缀表达式转后缀表达式
for token in tokens:
if isinstance(token, int):
output.append(token)
elif token in '^*/+-':
while stack and stack[-1] != '(' and priority[token] <= priority[stack[-1]]:
output.append(stack.pop())
stack.append(token)
elif token == '(':
stack.append(token)
elif token == ')':
while stack and stack[-1] != '(':
output.append(stack.pop())
stack.pop()
while stack:
output.append(stack.pop())
# 计算后缀表达式
stack = []
for token in output:
if isinstance(token, int):
stack.append(token)
elif token in '+-*/^':
op2 = stack.pop()
op1 = stack.pop()
if token == '+':
stack.append(op1 + op2)
elif token == '-':
stack.append(op1 - op2)
elif token == '*':
stack.append(op1 * op2)
elif token == '/':
stack.append(op1 // op2)
elif token == '^':
stack.append(op1 ** op2)
return stack[0]
# 输出结果
result = calculate(expr)
print(result)
```
假设测试数据文件in.txt中的算式为:"2+3*4-(5-6)",运行程序后输出的结果为:13
相关推荐
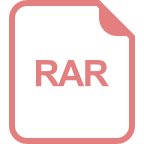
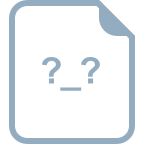
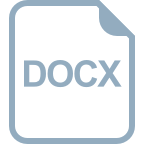














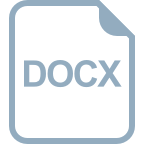