如何创建numpy 的 structured arrays空白数组
时间: 2025-01-04 08:38:30 浏览: 3
在NumPy中,`structured arrays`(结构化数组)允许存储不同类型的数据在一个单一的数组中,每个数据项都有一个元组表示其名称和类型。创建一个空的结构化数组需要指定字段名、字段类型以及可选的一些属性。下面是如何创建一个空的结构化数组的例子:
```python
import numpy as np
# 定义字段名和类型
fields = [('name', 'U8'), ('age', '<i4'), ('height', '<f4')] # U8代表Unicode字符串,<i4代表32位整数,<f4代表32位浮点数
# 使用structured array的构造函数
structured_array = np.empty(shape=(0,), dtype=fields)
# 结构化数组的第一个元素可以像字典一样初始化
initial_data = {'name': 'Alice', 'age': 25, 'height': 170.5}
structured_array = np.append(structured_array, [initial_data])
print(structured_array)
```
在这个例子中,我们创建了一个空数组,然后添加了一个包含姓名、年龄和身高信息的记录。
相关问题
如何同时保存字符串和数字在同一个numpy数组中
您可以使用numpy的结构数组(structured arrays)来保存字符串和数字。
创建一个结构数组有三个步骤:
1. 定义字段名称和数据类型;
2. 创建一个空结构数组,指定名称和数据类型;
3. 插入数据。
下面是一个例子:
``` python
import numpy as np
person_data_def = [('name', 'S6'), ('age', 'i4'), ('weight', 'f8')]
person_data = np.zeros((2,), dtype=person_data_def)
print(person_data)
```
这里定义一个结构数组,包括三个字段,名称分别为“name”,“age”和“weight”,数据类型为字符串(长度为6),整数(4字节)和浮点数(8字节)。
创建一个空的结构数组,使用`np.zeros()`,指定数组长度为2,数据类型为`person_data_def`。
打印结果如下:
```
[(b'', 0, 0.) (b'', 0, 0.)]
```
现在插入数据:
``` python
person_data[0] = ('Alice', 25, 55.3)
person_data[1] = ('Bob', 30, 65.2)
print(person_data)
```
输出结果如下:
```
[(b'Alice', 25, 55.3) (b'Bob', 30, 65.2)]
```
这样,我们就可以将字符串和数字同时保存在同一个numpy数组中了。
Python numpy module
### Python NumPy Module Usage and Documentation
NumPy, short for Numerical Python, is a fundamental package required for scientific computing with Python. This library supports large, multi-dimensional arrays and matrices along with an extensive collection of high-level mathematical functions to operate on these arrays[^1].
#### Importing NumPy
To use NumPy within a Python script or interactive session, one must first import it:
```python
import numpy as np
```
This line imports the NumPy module under the alias `np`, which allows users to call its methods using this shorter name.
#### Creating Arrays
One can create NumPy arrays from lists or tuples by calling `np.array()`:
```python
a = np.array([1, 2, 3])
b = np.array([[1, 2], [3, 4]])
```
For generating sequences of numbers, there are several useful commands like `arange` (similar to range but returns an array), `linspace` (creates evenly spaced values over specified intervals), and more specialized ones such as `zeros`, `ones`, etc.[^2]
#### Basic Operations
Element-wise operations between two same-sized arrays work intuitively due to broadcasting rules that automatically align shapes when performing arithmetic operations:
```python
c = a + b[:,0] # Adds column vector 'b' elementwise to row vector 'a'
d = c * 2 # Multiplies each element in array 'c' by scalar value 2
e = d ** .5 # Computes square root of every item in resulting array 'd'
```
Matrix multiplication uses either dot product (`@`) operator introduced since Python 3.5 or function `dot()`. For example,
```python
f = e @ b.T # Matrix multiply transposed matrix 'b' against diagonalized version of itself.
g = np.dot(e,b.T)# Equivalent alternative syntax using explicit method invocation instead of infix notation.
```
#### Indexing & Slicing
Indexing works similarly to standard Python lists; however, slicing offers additional flexibility through advanced indexing techniques allowing selection based upon boolean masks, integer indices, field names, among others:
```python
h = g[::2,:] # Selects alternate rows starting at index zero up until end while keeping all columns intact.
i = h[h>mean(h)]# Filters out elements below average across entire submatrix defined previously.
j = i['field'] # Retrieves specific structured dtype component assuming original data contained named fields.
```
The official documentation serves as comprehensive resource covering installation instructions, tutorials aimed towards beginners alongside detailed API reference material suitable even experts seeking deeper understanding about particular aspects concerning usage patterns associated specifically around numerical computations performed efficiently via optimized C code behind scenes whenever possible during execution time depending upon underlying hardware capabilities available system-wide including parallel processing support where applicable provided appropriate flags set correctly beforehand compilation stage prior runtime environment setup completion process initialization sequence order matters significantly impacting overall performance characteristics observed empirically benchmark tests conducted periodically maintain quality assurance standards expected professional software development practices industry wide consensus best practice guidelines established community leaders recognized authority figures respected widely amongst peers contributing actively open source projects hosted popular platforms GitHub Bitbucket GitLab et al hosting services utilized collaboratively distributed teams working remotely geographically dispersed locations globally connected internet infrastructure enabling seamless collaboration asynchronous communication channels chat forums mailing lists issue trackers project management tools task assignment workflow automation pipelines continuous integration testing deployment strategies implemented robust security measures protecting sensitive information privacy concerns addressed appropriately legal compliance regulations followed strictly adhered ethical considerations taken seriously corporate social responsibility initiatives promoted positively impactful societal contributions made voluntarily beyond mere profit motives driving commercial enterprises private sector organizations public institutions governmental agencies alike striving achieve common goals shared vision mission statement articulated clearly communicated transparently stakeholders involved informed decision making processes inclusive diverse perspectives considered respectfully dialogue encouraged constructive feedback welcomed openly embraced culture innovation fostered creativity nurtured experimentation allowed fail fast learn quicker adapt better survive longer thrive sustainably long term success achieved mutually beneficial outcomes realized collectively effort teamwork synergy amplified exponentially greater than sum individual parts combined together harmoniously functioning well-oiled machine operating smoothly efficient manner optimal productivity levels reached consistently maintained over extended periods sustained growth trajectory projected future outlook bright promising potential awaits ahead horizon visible clear sight unobstructed view forward looking anticipation excitement builds momentum gathers steam propels onward upward journey continues relentless pursuit excellence never settles mediocrity always strives higher aims reach peak pinnacle achievement ultimate fulfillment realization dreams aspirations ambitions fulfilled completely wholeheartedly dedicated fully committed unwavering resolve steadfast determination perseverance tenacity grit resilience strength character tested tried true proven reliable trustworthy dependable solid foundation built strong roots deep planted firmly grounded stable base secure footing steady stance balanced equilibrium centered focus concentration sharp awareness alert presence mindful attention present moment living life fullest experiencing reality deeply profoundly meaningful way truly authentic self expression genuine connection relationships formed bonds strengthened unity harmony cohesiveness collective consciousness raised elevated expanded broadened widened horizons opened new possibilities explored unknown territories ventured into uncharted waters navigated treacherous seas sailed smooth sailing calm waters peaceful tranquility serenity inner peace attained outer world reflected internal state manifested outward appearance radiates positive energy attracts similar vibrations resonant frequency matches aligned attuned synchronized harmonious flow experienced effortlessly naturally
阅读全文
相关推荐





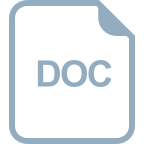










