爬取微博国羽苏杯三连冠新闻评论的代码
时间: 2023-09-06 20:05:16 浏览: 64
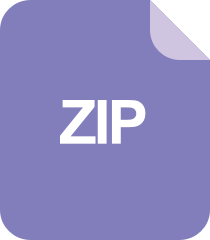
weibo_spider_spider_爬取微博_爬取微博评论_微博_weibospider_

### 回答1:
以下是基于 Python 的爬虫代码,使用 requests 和 BeautifulSoup 库进行网页内容的抓取和解析:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头部信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 构造需要爬取的页面URL链接
url = 'https://weibo.com/ttarticle/p/show?id=2309404635212305274774'
# 发送GET请求,获取网页内容
response = requests.get(url, headers=headers)
# 解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 查找评论区的 HTML 元素
comment_list = soup.find_all('div', class_='list_li S_line1 clearfix')
# 遍历评论区的 HTML 元素,提取评论内容并输出
for comment in comment_list:
content = comment.find('div', class_='WB_text W_f14').text.strip()
print(content)
```
注意:请遵守网络爬虫相关规定和法律法规,不要对网站造成过度的访问压力,以免引起不必要的麻烦。
### 回答2:
要爬取微博国羽苏杯三连冠新闻评论,可以使用Python编写代码实现。
首先,需要安装相关的Python库。可以使用pip命令安装beautifulsoup4和requests库。
```python
pip install beautifulsoup4
pip install requests
```
接下来,导入所需的库。
```python
import requests
from bs4 import BeautifulSoup
```
然后,构建请求链接,通过requests库发送GET请求获取网页内容。
```python
url = 'https://weibo.com/***' # 替换为微博新闻链接
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/92.0.4515.107 Safari/537.36'
}
response = requests.get(url, headers=headers)
```
接下来,使用BeautifulSoup库解析网页内容,找到评论部分的HTML元素。
```python
soup = BeautifulSoup(response.text, 'html.parser')
comment_divs = soup.find_all('div', class_='WB_text')
```
最后,遍历评论部分的HTML元素,提取评论内容并输出。
```python
for comment in comment_divs:
print(comment.text.strip())
```
以上就是简单的爬取微博国羽苏杯三连冠新闻评论的代码。请注意,爬取微博内容可能存在法律风险,请合法合规使用爬虫代码。
### 回答3:
爬取微博国羽苏杯三连冠新闻评论的代码可以使用Python编程语言来实现。下面是一个简单的代码示例:
```python
import requests
from bs4 import BeautifulSoup
def get_weibo_comments():
url = "https://m.weibo.cn/api/comments/show?id=4676648420806876&page=1"
headers = {
'User-Agent': 'Mozilla/5.0',
'Referer': 'https://m.weibo.cn/detail/4676648420806876'
}
comments = []
# 发送请求获取第一页评论
response = requests.get(url, headers=headers)
if response.status_code == 200:
json_data = response.json()
if json_data['ok'] == 1:
for comment in json_data['data']:
comments.append(comment['text'])
# 获取剩余页评论
page = 2
while True:
url = f"https://m.weibo.cn/api/comments/show?id=4676648420806876&page={page}"
response = requests.get(url, headers=headers)
if response.status_code == 200:
json_data = response.json()
if json_data['ok'] == 1:
for comment in json_data['data']:
comments.append(comment['text'])
page += 1
else:
break
return comments
# 调用函数获取评论并打印
comments = get_weibo_comments()
for comment in comments:
print(comment)
```
以上代码中,通过发送HTTP请求获取指定微博评论的JSON数据,并解析出评论内容保存到一个列表中。该代码模拟浏览器发送请求,需要设置`User-Agent`和`Referer`的请求头,以便正确获取数据。通过不断翻页,直到没有更多评论为止,获取所有评论内容,并将其打印出来。
需要注意的是,具体的微博评论API URL可能会有变化,上述代码中的URL仅为示例。在实际使用时,请使用正确的URL或根据需要进行参数的修改。
阅读全文
相关推荐
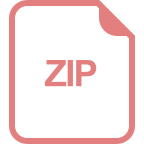
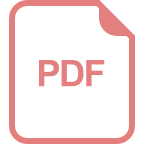















