请帮我用c++生成一段面向对象的贪吃蛇代码
时间: 2024-03-15 07:45:03 浏览: 14
好的,以下是一个简单的面向对象的贪吃蛇游戏的C++代码,希望能够帮到您:
```c++
#include <iostream>
#include <vector>
#include <conio.h>
#include <windows.h>
using namespace std;
const int Width = 20, Height = 20;
int Score = 0;
class Point {
public:
int x, y;
Point(int _x = 0, int _y = 0) : x(_x), y(_y) {}
};
class Snake {
public:
vector<Point> body;
Point dir;
Snake(Point head = Point(0, 0)) {
body.push_back(head);
dir = Point(1, 0);
}
void Move() {
for (int i = body.size() - 1; i > 0; i--) {
body[i].x = body[i - 1].x;
body[i].y = body[i - 1].y;
}
body[0].x += dir.x;
body[0].y += dir.y;
}
bool IsDead() {
if (body[0].x < 0 || body[0].x >= Width || body[0].y < 0 || body[0].y >= Height)
return true;
for (int i = 1; i < body.size(); i++) {
if (body[0].x == body[i].x && body[0].y == body[i].y)
return true;
}
return false;
}
void AddBody() {
int x = body[body.size() - 1].x - dir.x;
int y = body[body.size() - 1].y - dir.y;
body.push_back(Point(x, y));
}
};
class Food {
public:
Point pos;
void Spawn() {
pos.x = rand() % Width;
pos.y = rand() % Height;
}
};
class Playground {
public:
Snake snake;
Food food;
void Init() {
snake = Snake(Point(Width / 2, Height / 2));
food.Spawn();
}
void Draw() {
system("cls");
for (int i = 0; i < Width + 2; i++)
cout << "#";
cout << endl;
for (int i = 0; i < Height; i++) {
cout << "#";
for (int j = 0; j < Width; j++) {
if (i == snake.body[0].y && j == snake.body[0].x)
cout << "O";
else if (i == food.pos.y && j == food.pos.x)
cout << "F";
else {
bool flag = false;
for (int k = 1; k < snake.body.size(); k++) {
if (i == snake.body[k].y && j == snake.body[k].x) {
flag = true;
cout << "o";
break;
}
}
if (!flag)
cout << " ";
}
}
cout << "#";
cout << endl;
}
for (int i = 0; i < Width + 2; i++)
cout << "#";
cout << endl;
cout << "Score: " << Score << endl;
}
void Input() {
if (_kbhit()) {
char ch = _getch();
if (ch == 'w' && snake.dir.y != 1)
snake.dir = Point(0, -1);
if (ch == 's' && snake.dir.y != -1)
snake.dir = Point(0, 1);
if (ch == 'a' && snake.dir.x != 1)
snake.dir = Point(-1, 0);
if (ch == 'd' && snake.dir.x != -1)
snake.dir = Point(1, 0);
}
}
void Update() {
snake.Move();
if (snake.IsDead()) {
cout << "Game Over!" << endl;
Sleep(2000);
exit(0);
}
if (snake.body[0].x == food.pos.x && snake.body[0].y == food.pos.y) {
Score += 10;
snake.AddBody();
food.Spawn();
}
}
};
int main() {
Playground playground;
playground.Init();
while (true) {
playground.Draw();
playground.Input();
playground.Update();
Sleep(100);
}
return 0;
}
```
以上代码实现了一个简单的贪吃蛇游戏,其中使用了面向对象的编程思想,将蛇、食物和游戏场地等元素进行了封装。具体来说:
1. 定义了一个Point类来表示坐标,在Snake和Food类中使用。
2. 定义了一个Snake类来表示蛇,在其中包含了蛇的身体、方向和移动等属性和方法。
3. 定义了一个Food类来表示食物,在其中包含了食物的位置和生成等属性和方法。
4. 定义了一个Playground类来表示游戏场地,在其中包含了蛇、食物和游戏初始化、绘制、输入和更新等属性和方法。
5. 在主函数中,首先创建了一个Playground实例,并进入了游戏循环,不断更新游戏状态并将其绘制到屏幕上。
6. 在游戏循环中,添加了键盘事件监听器,用于控制蛇的方向,并进行了碰撞检测,判断蛇是否吃到了食物。如果吃到了食物,则让蛇生长,并重新生成一个新的食物。
总的来说,以上代码实现了一个简单的贪吃蛇游戏,具有一定的可玩性,同时也体现了面向对象的编程思想。
相关推荐
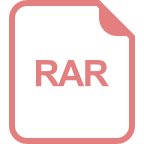














