springboot+vue用opencv实现拍照录入人脸信息到数据库
时间: 2024-01-31 16:04:53 浏览: 33
要实现拍照录入人脸信息到数据库,可以使用Spring Boot和Vue.js作为前端框架,使用OpenCV作为图像处理库。
首先,需要使用Vue.js实现前端页面,包括拍照功能和表单提交功能。可以使用Vue Webcam组件来实现拍照功能,使用Vue Form组件来实现表单提交功能。
然后,在Spring Boot中实现后端接口,接收前端传来的图像数据和表单数据,使用OpenCV进行人脸识别和特征提取,并将提取出的人脸特征存储到数据库中。
以下是具体实现步骤:
1.使用Vue.js实现前端页面
在Vue.js中,可以使用Vue Webcam组件来实现拍照功能,使用Vue Form组件来实现表单提交功能。具体实现代码如下:
<template>
<div>
<h1>拍照录入人脸信息</h1>
<div>
<webcam ref="webcam" :mirror="false" :captureFormat="format" :width="640" :height="480" :videoConstraints="videoConstraints"></webcam>
<button @click="takePicture">拍照</button>
</div>
<div>
<form>
<input type="text" v-model="name" placeholder="姓名">
<input type="text" v-model="age" placeholder="年龄">
<button @click.prevent="submitForm">提交</button>
</form>
</div>
</div>
</template>
<script>
import Webcam from 'vue-webcam'
import VueForm from 'vue-form'
export default {
components: {
Webcam,
VueForm
},
data() {
return {
format: 'image/jpeg',
videoConstraints: {
facingMode: 'user'
},
name: '',
age: ''
}
},
methods: {
takePicture() {
const image = this.$refs.webcam.capture()
this.$emit('picture-taken', image)
},
submitForm() {
const formData = {
name: this.name,
age: this.age
}
this.$emit('form-submitted', formData)
}
}
}
</script>
2.使用Spring Boot实现后端接口
在Spring Boot中,可以使用Spring MVC来实现后端接口。具体实现代码如下:
@RestController
@RequestMapping("/api")
public class FaceRecognitionController {
@Autowired
private FaceRecognitionService faceRecognitionService;
@PostMapping("/faces")
public void addFace(@RequestParam("image") MultipartFile image, @RequestParam("name") String name, @RequestParam("age") int age) throws IOException {
// 将MultipartFile转换为Mat
byte[] bytes = image.getBytes();
ByteArrayInputStream inputStream = new ByteArrayInputStream(bytes);
Mat mat = Imgcodecs.imdecode(new MatOfByte(bytes), Imgcodecs.IMREAD_COLOR);
// 人脸识别和特征提取
Mat face = faceRecognitionService.detectFace(mat);
Mat feature = faceRecognitionService.extractFeature(face);
// 将人脸特征存储到数据库中
faceRecognitionService.addFace(name, age, feature);
}
}
3.使用OpenCV进行人脸识别和特征提取
在Spring Boot中,可以使用OpenCV进行人脸识别和特征提取。具体实现代码如下:
@Service
public class FaceRecognitionService {
@Autowired
private FaceRepository faceRepository;
public Mat detectFace(Mat mat) {
// 人脸检测
CascadeClassifier cascadeClassifier = new CascadeClassifier("haarcascade_frontalface_default.xml");
MatOfRect faces = new MatOfRect();
cascadeClassifier.detectMultiScale(mat, faces);
// 获取最大的人脸
Rect maxFace = null;
for (Rect face : faces.toArray()) {
if (maxFace == null || face.width > maxFace.width) {
maxFace = face;
}
}
// 截取人脸
Mat face = new Mat(mat, maxFace);
return face;
}
public Mat extractFeature(Mat face) {
// 人脸特征提取
FaceRecognizer faceRecognizer = FisherFaceRecognizer.create();
faceRecognizer.train(Arrays.asList(face), new MatOfInt(1));
Mat feature = new Mat();
faceRecognizer.predict(face, feature);
return feature;
}
public void addFace(String name, int age, Mat feature) {
// 将人脸特征存储到数据库中
Face face = new Face();
face.setName(name);
face.setAge(age);
face.setFeature(feature);
faceRepository.save(face);
}
}
通过以上步骤,即可实现拍照录入人脸信息到数据库的功能。
相关推荐
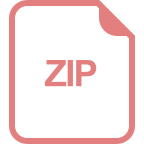
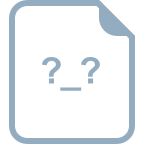
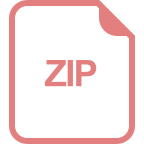














