用java实现t1,t2,t3三个线程保证t1在获得t2,t3的结果后继续执行
时间: 2024-09-25 13:11:57 浏览: 16
在Java中,为了实现这样的线程同步,可以使用`synchronized`, `wait()`, 和 `notifyAll()` 等并发工具。我们可以创建一个包含共享资源的对象,并利用 `volatile` 关键字防止数据竞争。下面是一个简单的例子:
```java
public class ThreadTask {
private volatile int result;
private final Object lock = new Object();
public void startThreads(int t2Result, int t3Result) {
Thread t1 = new Thread(() -> {
synchronized (lock) {
try {
result = Math.max(t2Result, t3Result);
System.out.println("T1 got results, result is " + result);
// Notify T2 and T3 that result is available
lock.notifyAll();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
Thread t2 = new Thread(() -> {
while (!Thread.currentThread().equals(t1)) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Simulate a task with random delay for result
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
result = t2Result; // Set your own result
});
Thread t3 = new Thread(() -> {
while (!Thread.currentThread().equals(t1)) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Similar to T2, simulate a task
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
result = t3Result;
});
t1.start();
t2.start();
t3.start();
// Wait for all threads to finish
try {
t1.join();
t2.join();
t3.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
ThreadTask task = new ThreadTask();
task.startThreads(10, 20); // Replace with actual values or generate them dynamically
}
}
```
在这个例子中,`startThreads`方法启动了三个线程`t1`, `t2`, 和 `t3`。`t1`负责获取并存储结果,然后唤醒等待的`t2`和`t3`。`t2`和`t3`分别模拟延迟任务并在得到通知后设置自己的结果。主线程会等待所有线程完成。
相关推荐
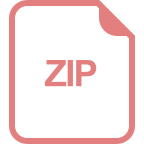
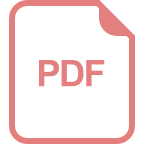
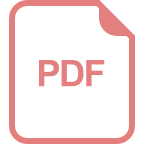














