现在有T1 打印(1,2,3)、T2(4,5,6)、T3(7,8,9)三个线程,你怎样保证T2在T1执行完后执行,T3在T2执行完后执行?请用Java代码实现
时间: 2024-03-03 22:50:05 浏览: 119
可以使用Java中的线程同步机制来实现三个线程的执行顺序,以下是Java代码示例:
```java
public class PrintThread implements Runnable {
private static Object lock = new Object();
private int start;
private int end;
public PrintThread(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public void run() {
synchronized (lock) {
for (int i = start; i <= end; i++) {
System.out.print(i + " ");
}
System.out.println();
lock.notify(); // 唤醒其它线程
}
}
public static void main(String[] args) {
PrintThread t1 = new PrintThread(1, 3);
PrintThread t2 = new PrintThread(4, 6);
PrintThread t3 = new PrintThread(7, 9);
try {
synchronized (lock) {
new Thread(t1).start();
lock.wait(); // 让t1先执行
new Thread(t2).start();
lock.wait(); // 让t2先执行
new Thread(t3).start();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
在主线程中创建三个打印线程t1、t2、t3。使用synchronized关键字锁定一个共享对象,确保三个线程中只有一个线程能够执行。在主线程中启动t1线程并等待它执行完毕,然后启动t2线程并等待它执行完毕,最后启动t3线程。在每个线程的run方法中,先使用synchronized关键字锁定共享对象,然后打印对应的数字,最后唤醒其它线程。
阅读全文
相关推荐






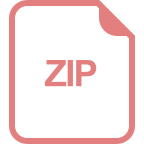







