求数组中三个数的最小公倍数
时间: 2024-06-13 16:09:00 浏览: 171
以下是求数组中三个数的最小公倍数的Python代码:
```python
def gcd(a, b):
if b == 0:
return a
else:
return gcd(b, a % b)
def lcm(a, b):
return a * b // gcd(a, b)
def lcm_of_array(arr):
n = len(arr)
ans = arr[0]
for i in range(1, n):
ans = lcm(ans, arr[i])
return ans
arr = [2, 3, 4]
print("The LCM of the array is:", lcm_of_array(arr)) # 输出:The LCM of the array is: 12
```
首先,我们需要定义一个求两个数最大公约数的函数gcd,以及一个求两个数最小公倍数的函数lcm。然后,我们遍历数组中的每个数,将它们的最小公倍数依次求出来,最后得到的就是整个数组的最小公倍数。在上面的代码中,我们以数组[2, 3, 4]为例,最终得到的最小公倍数为12。
相关问题
计算整形数组a中相邻两个元素的最大公约数,最小公倍数,其中最大公约数存入数组b中,最小公倍数存入数组c中,C语言,如何写,不用函数封装的形式写,要完整,用3种方法
方法一:
```c
#include <stdio.h>
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
int lcm(int a, int b) {
return a * b / gcd(a, b);
}
int main() {
int a[] = {6, 9, 12, 15, 18};
int n = 5;
int b[n - 1], c[n - 1];
for (int i = 0; i < n - 1; i++) {
b[i] = gcd(a[i], a[i + 1]);
c[i] = lcm(a[i], a[i + 1]);
}
for (int i = 0; i < n - 1; i++) {
printf("%d ", b[i]);
}
printf("\n");
for (int i = 0; i < n - 1; i++) {
printf("%d ", c[i]);
}
printf("\n");
return 0;
}
```
方法二:
```c
#include <stdio.h>
int gcd(int a, int b) {
while (b != 0) {
int t = b;
b = a % b;
a = t;
}
return a;
}
int lcm(int a, int b) {
return a * b / gcd(a, b);
}
int main() {
int a[] = {6, 9, 12, 15, 18};
int n = 5;
int b[n - 1], c[n - 1];
for (int i = 0; i < n - 1; i++) {
b[i] = gcd(a[i], a[i + 1]);
c[i] = lcm(a[i], a[i + 1]);
}
for (int i = 0; i < n - 1; i++) {
printf("%d ", b[i]);
}
printf("\n");
for (int i = 0; i < n - 1; i++) {
printf("%d ", c[i]);
}
printf("\n");
return 0;
}
```
方法三:
```c
#include <stdio.h>
int gcd(int a, int b) {
while (a != b) {
if (a > b) {
a -= b;
} else {
b -= a;
}
}
return a;
}
int lcm(int a, int b) {
return a * b / gcd(a, b);
}
int main() {
int a[] = {6, 9, 12, 15, 18};
int n = 5;
int b[n - 1], c[n - 1];
for (int i = 0; i < n - 1; i++) {
b[i] = gcd(a[i], a[i + 1]);
c[i] = lcm(a[i], a[i + 1]);
}
for (int i = 0; i < n - 1; i++) {
printf("%d ", b[i]);
}
printf("\n");
for (int i = 0; i < n - 1; i++) {
printf("%d ", c[i]);
}
printf("\n");
return 0;
}
```
使用C语言找两个数的最小公倍数
在C语言中,计算两个正整数的最小公倍数(LCM)通常涉及到求它们的最大公约数(GCD),因为LCM可以通过乘以两数的GCD来得到。以下是欧几里得算法(辗转相除法)用于求解GCD的示例[^1]:
```c
// 定义求最大公约数的函数
int gcd(int a, int b) {
if (b == 0) return a;
return gcd(b, a % b);
}
// 求两个数的最小公倍数
int lcm(int a, int b) {
return (a * b) / gcd(a, b); // 最小公倍数等于两数之积除以其最大公约数
}
```
要计算任意两个数的最小公倍数,只需调用上述`lcm()`函数,比如`lcm(15, 25)`。对于多于两个的数,可以递归地应用此方法[^2],如计算5个数的最小公倍数:
```c
#include <stdio.h>
// 基于已知两个数的情况求三个数的最小公倍数
void find_lcm_for_three(int* arr, int n) {
int temp = lcm(arr[n - 2], arr[n - 1]);
arr[n - 1] = temp;
}
// 求五个数的最小公倍数
void find_lcm_for_five(int* arr, int n) {
for (int i = 3; i < n; ++i)
find_lcm_for_three(arr, i + 1);
}
int main() {
int numbers[] = {10, 20, 30, 40, 50}; // 示例数组
int n = sizeof(numbers) / sizeof(numbers[0]);
find_lcm_for_five(numbers, n);
printf("The least common multiple of the five numbers is: %d\n", numbers[n - 1]);
return 0;
}
```
阅读全文
相关推荐




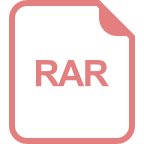

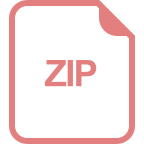









