python三维向量变二维向量
时间: 2023-07-08 14:48:31 浏览: 108
将三维向量降至二维向量同样可以使用主成分分析(PCA)的方法。具体来说,我们可以把三维向量看作是一个1x3的矩阵,然后使用PCA将其降至1x2的矩阵。下面是一个简单的示例代码:
```python
from sklearn.decomposition import PCA
import numpy as np
# 生成随机三维向量
x = np.random.rand(1, 3)
# 实例化PCA模型,将向量降至二维
pca = PCA(n_components=2)
x_reduced = pca.fit_transform(x)
# 输出降维后的向量
print(x_reduced.shape) # (1, 2)
```
在上面的代码中,我们生成了一个1x3的随机向量x,然后使用PCA将其降至1x2的向量x_reduced。需要注意的是,由于PCA只能处理二维以上的数据,因此我们需要将1x3的向量转化为1x3的矩阵。
相关问题
python二维向量叉乘
Python中,可以使用NumPy库来进行向量的叉乘运算。具体实现方法如下:
```python
import numpy as np
# 定义两个二维向量
a = np.array([1, 2])
b = np.array([3, 4])
# 计算叉乘
c = np.cross(a, b)
# 输出结果
print(c)
```
运行结果为:
```
-2
```
上述代码中,我们首先导入了NumPy库,然后定义了两个二维向量a和b。接着,使用np.cross()函数计算了这两个向量的叉乘,并将结果保存在变量c中。最后,使用print()函数输出了结果。
需要注意的是,向量的叉乘运算只适用于三维向量,因此在进行二维向量的叉乘时,需要将其补齐为三维向量,其中第三个分量为0。
python 三维点 主成分 特征向量
Python中可以使用NumPy库来处理三维点和进行主成分分析。
三维点指的是具有三个坐标轴的点,通常用(x, y, z)表示。
主成分分析是一种常用的统计方法,用于分析多维数据中的主要特征。在三维点的情况下,主成分分析可以用于确定数据点的主要方向和分布情况。
在Python中,可以使用NumPy库的函数来进行主成分分析,其中最常用的是numpy.linalg.eig()函数。这个函数可以计算矩阵的特征值和特征向量。
特征向量是矩阵在进行线性变换时所指向的方向,而特征值则表示该特征向量在该方向上的缩放倍数。在三维点的情况下,特征向量是表示主要方向的单位向量,而特征值则表示数据在该方向上的重要程度。
在主成分分析中,首先需要将三维点集表示为一个矩阵,然后通过对该矩阵进行特征值分解,可以得到特征值和特征向量。特征值越大,表示该方向上的数据变化越大,对应的特征向量就是主成分。
总而言之,Python中可以使用NumPy库来处理三维点和进行主成分分析。通过计算特征值和特征向量,可以确定数据点的主要方向和分布情况。特征向量是主要方向的单位向量,而特征值表示数据在该方向上的重要程度。这些信息可以用于数据分析和可视化等领域。
相关推荐
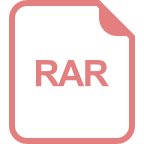












