python定义三维向量类
时间: 2023-09-28 10:03:22 浏览: 133
可以使用 Python 中的类来定义一个三维向量类。下面是一个简单的例子:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self):
return f"({self.x}, {self.y}, {self.z})"
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
return Vector3D(self.x * other, self.y * other, self.z * other)
def __rmul__(self, other):
return Vector3D(self.x * other, self.y * other, self.z * other)
def dot(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross(self, other):
return Vector3D(self.y * other.z - self.z * other.y,
self.z * other.x - self.x * other.z,
self.x * other.y - self.y * other.x)
def magnitude(self):
return (self.x ** 2 + self.y ** 2 + self.z ** 2) ** 0.5
```
这个类包含了三个属性:x、y、z,表示三维向量的三个分量。同时,我们还定义了 __repr__ 方法,用于将向量对象转换为字符串形式进行输出。
在类中,我们还定义了 __add__、__sub__、__mul__、__rmul__ 方法,用于向量的加减乘除等运算。同时,我们还定义了 dot 和 cross 方法,分别表示向量的点积和叉积运算。最后,我们还定义了 magnitude 方法,用于计算向量的模长。
使用这个类,我们可以方便地进行三维向量的运算。例如:
```python
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
# 向量加法
v3 = v1 + v2
print(v3) # 输出:(5, 7, 9)
# 向量点积
dot_product = v1.dot(v2)
print(dot_product) # 输出:32
# 向量叉积
cross_product = v1.cross(v2)
print(cross_product) # 输出:(-3, 6, -3)
# 向量模长
magnitude = v1.magnitude()
print(magnitude) # 输出:3.7416573867739413
```
相关推荐
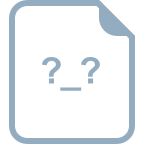














