Overriding Superclass Methods
时间: 2024-11-25 18:31:37 浏览: 28
"覆盖超类方法"通常是指在面向对象编程中,当一个子类继承自一个父类时,它有权限提供对父类中某些方法的不同实现。这种行为称为方法重写(Method Override)。具体来说:
1. **目的**:通过重写,子类可以修改或增强其父类的行为,使其适应更特定的场景或需求。这增加了程序的灵活性和可扩展性。
2. **语法**:在Java中,使用 `override` 关键字声明方法重写,子类方法的名称、返回类型、参数列表和访问修饰符都必须与父类方法完全匹配。例如:
```java
class Parent {
public void printMessage() {
System.out.println("Parent message");
}
}
class Child extends Parent {
@Override
public void printMessage() {
System.out.println("Child message");
}
}
```
子类`Child`中的`printMessage()`会覆盖`Parent`的同名方法。
3. **注意事项**:
- 覆盖方法时,权限不能降低(如从`public`变为`private`)。
- 如果子类不重写父类的方法,那么默认继承父类的方法实现。
相关问题
Design and implement circle class and cylinder class. class circle must be the superclass of class cylinder. class circle cannot have any superclass except the ultimate superclass object. when instantiating the two classes , circles and cylinders can be made and stored in an array list as shown below: Arraylist<Circle> shapes=new Arraylist <Circle>(); shapes.add (new Circle());shapes.add(new Circle(5));shapes.add(new Cylinder());shapes.add(new Cylinder(10,5));for (Circle next:shapes){ System.out.println(next);} Implement both classes by including only the following members in each of the classes:instance variables,overloading consructors,getters,setters,overriding toString,overriding equals
Sure, here's an implementation of the Circle and Cylinder classes according to the requirements you specified:
```
import java.util.Objects;
public class Circle {
private double radius;
public Circle() {
this.radius = 1.0;
}
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public String toString() {
return "Circle with radius: " + radius;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Circle circle = (Circle) o;
return Double.compare(circle.radius, radius) == 0;
}
@Override
public int hashCode() {
return Objects.hash(radius);
}
}
public class Cylinder extends Circle {
private double height;
public Cylinder() {
super();
this.height = 1.0;
}
public Cylinder(double height, double radius) {
super(radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public String toString() {
return "Cylinder with height: " + height + " and radius: " + getRadius();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
Cylinder cylinder = (Cylinder) o;
return Double.compare(cylinder.height, height) == 0;
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), height);
}
}
```
In this implementation, the Circle class has an instance variable for the radius and provides two constructors (one with a default radius of 1.0 and one that takes a specific radius), getters and setters for the radius, and overrides the toString and equals methods.
The Cylinder class extends the Circle class and adds an instance variable for the height as well as its own constructors, getters and setters, and overrides of toString and equals. Note that the Cylinder class calls the constructor of the Circle class using `super(radius)` to set the radius value inherited from the superclass.
You can use these classes to create circles and cylinders and store them in an ArrayList as follows:
```
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Circle> shapes = new ArrayList<Circle>();
shapes.add(new Circle());
shapes.add(new Circle(5));
shapes.add(new Cylinder());
shapes.add(new Cylinder(10, 5));
for (Circle next : shapes) {
System.out.println(next);
}
}
}
```
This code creates an ArrayList of Circle objects, adds four different shapes to it (two circles and two cylinders), and then prints out the toString representation of each shape. The output will look something like this:
```
Circle with radius: 1.0
Circle with radius: 5.0
Cylinder with height: 1.0 and radius: 1.0
Cylinder with height: 10.0 and radius: 5.0
```
Describe these two approaches to polymorphism in Java: Overloading and Overriding. Give an example of each.
Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as if they were objects of the same class. In Java, there are two common ways to implement polymorphism: Overloading and Overriding.
1. Overloading: This occurs when multiple methods have the same name but different parameters. The method that is called depends on the type and number of arguments passed to it. Overloading is a way to provide different implementations of the same method for different types of input.
Example:
```
public class MathOperations {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
```
In the example above, there are two methods called `add`, but one takes two `int` parameters, and the other takes two `double` parameters. When the `add` method is called, the appropriate method is selected based on the parameters passed to it.
2. Overriding: This occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The subclass method must have the same name, return type, and parameters as the superclass method. The subclass method can also have a more specific access modifier than the superclass method.
Example:
```
public class Shape {
public void draw() {
System.out.println("Drawing a shape.");
}
}
public class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a circle.");
}
}
```
In the example above, the `Circle` class overrides the `draw` method of its superclass `Shape`. When the `draw` method is called on a `Circle` object, the `Circle` implementation of the method is executed instead of the `Shape` implementation.
阅读全文
相关推荐
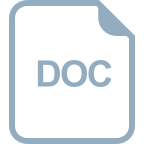
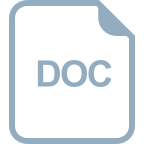
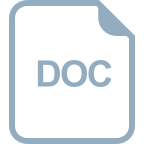

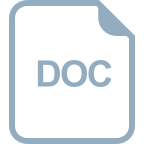
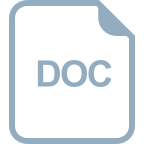
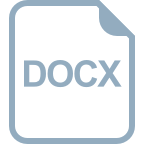
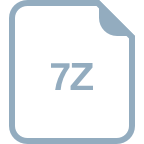
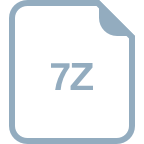
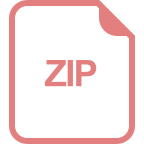
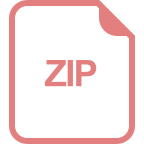
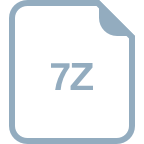