python中调用外部函数
时间: 2023-11-12 09:06:14 浏览: 75
在Python中调用外部函数,通常需要使用import语句将外部函数导入到当前的Python脚本中,然后即可使用其中的函数。
例如,如果需要调用外部模块中的函数,可以使用以下语句:
```python
import module_name
# 调用外部模块中的函数
module_name.function_name()
```
如果需要调用外部库中的函数,可以使用以下语句:
```python
import library_name
# 调用外部库中的函数
library_name.function_name()
```
如果需要调用外部可执行文件中的函数,可以使用以下语句:
```python
import subprocess
# 调用外部可执行文件中的函数
subprocess.call(['executable_name', 'arg1', 'arg2'])
```
其中,`executable_name`表示外部可执行文件的名称,`arg1`和`arg2`表示传递给外部函数的参数。
相关问题
如何在Python中调用嵌套函数?
在Python中,你可以直接通过函数名来调用嵌套函数。这里以`ex.py`文件为例,如果有一个嵌套函数`nested_function`,你可以这样调用:
```python
def outer_function():
def nested_function():
# 这里是你嵌套函数的具体代码
# 调用嵌套函数
nested_function()
# 使用thread模块运行这个外部函数(如果你的目的是并发执行)
import thread
thread.start_new_thread(outer_function, ()) # 注意:在Robot Framework中,可能需要稍有不同的方式来启动线程,具体取决于你的设置。
```
要单独运行嵌套函数,通常不需要使用`thread`模块,因为嵌套函数可以作为独立的可调用对象存在。只需在其定义后直接调用即可:
```python
nested_result = nested_function() # 如果你想保存结果
nested_function() # 或者只是简单地执行它
```
如何在MainWindow(QMainWindow)中调用外部函数
要在MainWindow中调用外部函数,可以使用以下步骤:
1. 在MainWindow的构造函数中导入外部模块或文件,例如:
```python
from mymodule import myfunction
```
这将导入名为`myfunction`的函数,该函数定义在名为`mymodule`的模块中。
2. 在MainWindow中定义一个槽函数,该函数将作为GUI中的按钮或菜单项的回调函数。例如:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# Import external module
from mymodule import myfunction
# Create button to call external function
button = QPushButton("Call My Function", self)
button.clicked.connect(self.call_my_function)
def call_my_function(self):
# Call external function
myfunction()
```
在上面的示例中,我们定义了一个名为`call_my_function`的槽函数,该函数将在按钮被点击时被调用。该函数将调用之前导入的`myfunction`函数。
注意:如果你想调用的函数不是一个标准的Python模块或文件中的函数,而是一个在外部进程中运行的函数,那么你需要使用通信机制,例如进程间通信(IPC),来与该进程进行通信。
阅读全文
相关推荐
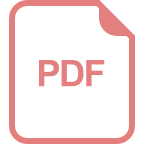

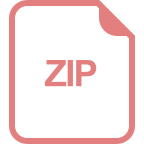

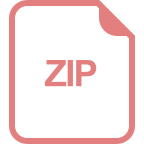
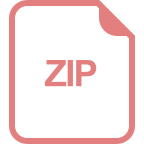
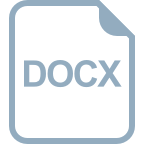
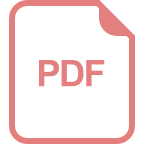
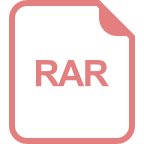
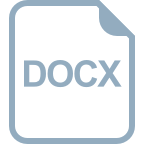
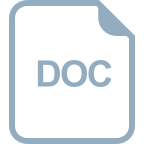
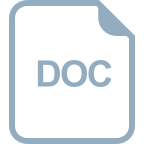
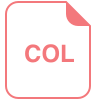

