提供一份同步FIFO的Verilog代码
时间: 2023-08-14 07:51:42 浏览: 53
Sure, here's an example of Verilog code for a synchronous FIFO (First-In-First-Out) implementation:
```verilog
module SyncFIFO (
input wire clk,
input wire rst,
input wire wr_en,
input wire rd_en,
input wire [DATA_WIDTH-1:0] data_in,
output wire [DATA_WIDTH-1:0] data_out,
output wire empty,
output wire full
);
parameter DEPTH = 16;
parameter DATA_WIDTH = 8;
reg [DATA_WIDTH-1:0] fifo [DEPTH-1:0];
reg [DEPTH-1:0] wr_ptr;
reg [DEPTH-1:0] rd_ptr;
reg [DEPTH-1:0] next_wr_ptr;
reg [DEPTH-1:0] next_rd_ptr;
reg [DATA_WIDTH-1:0] next_data_out;
reg empty_reg, full_reg;
always @(posedge clk or posedge rst) begin
if (rst) begin
wr_ptr <= 0;
rd_ptr <= 0;
empty_reg <= 1;
full_reg <= 0;
end else begin
wr_ptr <= next_wr_ptr;
rd_ptr <= next_rd_ptr;
empty_reg <= (wr_ptr == rd_ptr);
full_reg <= (next_wr_ptr == rd_ptr);
end
end
always @(posedge clk) begin
if (rst) begin
next_wr_ptr <= 0;
next_rd_ptr <= 0;
next_data_out <= 0;
end else begin
if (wr_en && ~full_reg) begin
next_wr_ptr <= wr_ptr + 1;
fifo[wr_ptr] <= data_in;
end else begin
next_wr_ptr <= wr_ptr;
end
if (rd_en && ~empty_reg) begin
next_rd_ptr <= rd_ptr + 1;
next_data_out <= fifo[rd_ptr];
end else begin
next_rd_ptr <= rd_ptr;
end
end
end
assign data_out = next_data_out;
assign empty = empty_reg;
assign full = full_reg;
endmodule
```
Please note that this is a simple implementation and might need modifications based on your specific requirements, such as clock frequency, data width, and depth of the FIFO.
相关推荐
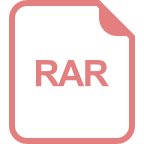














