python cv2 新建空白图片
时间: 2023-07-28 13:10:36 浏览: 115
你可以使用 OpenCV 库的 cv2 模块来创建一个空白图片。以下是创建一个 640x480 像素、黑色背景的空白图片的示例代码:
```python
import numpy as np
import cv2
# 创建一个黑色背景的空白图片
img = np.zeros((480, 640, 3), np.uint8)
# 显示图片
cv2.imshow("Blank Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们使用 numpy 库创建了一个 480x640 的空白图片,像素值全部为 0,即黑色背景。然后使用 cv2.imshow() 函数显示了这个图片。你可以根据自己的需要修改图片的大小和背景颜色。
相关问题
python新建空白dagaframe
要新建一个空白的 DataFrame,你需要导入 pandas 库并调用 DataFrame() 方法。以下是代码示例:
```python
import pandas as pd
df = pd.DataFrame()
```
这将创建一个没有行或列的空白 DataFrame。你可以通过添加列和行来填充它。
python word新建空白页
如果你想在 Python 中创建一个新的空白页,可以使用第三方库 `reportlab`。下面是一个简单的示例代码:
```python
from reportlab.pdfgen.canvas import Canvas
# 创建一个新的 PDF 文件
canvas = Canvas("new_blank_page.pdf")
# 在 PDF 文件中添加空白页
canvas.showPage()
# 保存 PDF 文件并关闭画布
canvas.save()
```
这将创建一个名为 `new_blank_page.pdf` 的新 PDF 文件,并向其中添加一个空白页。你可以根据需要修改文件名和路径。
相关推荐
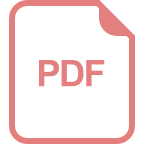












