class Account implements Runnable{ private int money; //修改方法 @Override public void run() { // TODO Auto-generated method stub } 给上代码写一个访问方法
时间: 2024-03-26 13:42:08 浏览: 15
以下是一个简单的访问方法,可以获取 Account 对象中的 money 属性:
```
public synchronized int getMoney() {
return money;
}
```
这里使用 synchronized 关键字来保证线程安全,确保在多线程环境下获取 money 属性的值是正确的。
相关问题
优化代码运用线程知识package D18; public class D18_1 { public static void main(String[] args) { // TODO Auto-generated method stub } } class Account implements Runnable{ private int money; //修改方法 //存钱 public void deposit(int amount) { money +=amount; System.out.println("存钱成功,现有"+money); } @Override public void run() { // TODO Auto-generated method stub deposit(100); } public int getMoney() { return money; } public void setMoney(int money) { this.money = money; } }
以下是对代码的优化,使用线程来实现存钱和查询余额的功能:
```
public class D18_1 {
public static void main(String[] args) throws InterruptedException {
Account account = new Account();
Thread depositThread = new Thread(() -> {
for (int i = 0; i < 10; i++) {
account.deposit(100);
}
});
Thread checkThread = new Thread(() -> {
for (int i = 0; i < 10; i++) {
System.out.println("当前余额为:" + account.getMoney());
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
depositThread.start();
checkThread.start();
depositThread.join();
checkThread.join();
}
}
class Account {
private int money;
public synchronized void deposit(int amount) {
money += amount;
System.out.println("存钱成功,现有" + money);
}
public synchronized int getMoney() {
return money;
}
}
```
这里使用了 synchronized 关键字来保证线程安全,同时使用了 join() 方法来保证查询余额的线程在存钱线程执行完毕后才开始运行,避免了查询余额时余额数值不正确的问题。
请编写一个java程序,创建两个线程,一个线程每隔1秒输出“hello,world”,输出10次,退出,一个线程每隔1秒输出“hi”,输出5次退出。public class ThreadHomework { public static void main(String[] args) { // TODO Auto-generated method stub T1 t1=new T1(); T2 t2=new T2(); //创建线程th1,th2,并开启线程 } } class T1 implements Runnable{ ////定义T1类,每隔1秒输出“hello,world”,输出10次。 } class T2 implements Runnable{ //定义T2类,每隔1秒输出“hi”,输出5次退出。 } 根据解释完善代码
以下是完整的Java程序代码:
```
public class ThreadHomework {
public static void main(String[] args) {
T1 t1 = new T1();
T2 t2 = new T2();
Thread th1 = new Thread(t1);
Thread th2 = new Thread(t2);
th1.start();
th2.start();
}
}
class T1 implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 10; i++) {
System.out.println("hello,world");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class T2 implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 5; i++) {
System.out.println("hi");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
在上面的代码中,我们定义了两个线程类T1和T2,它们都实现了Runnable接口并重写了run()方法。在T1类中,我们使用for循环输出了10次“hello,world”,并在每次输出后通过Thread.sleep()方法让线程休眠1秒钟,以达到每隔1秒输出一次的效果。在T2类中,我们同样使用for循环输出了5次“hi”,并在每次输出后让线程休眠1秒钟。
在ThreadHomework类的main()方法中,我们创建了两个线程对象t1和t2,并将它们传递给Thread类的构造方法来创建线程th1和th2。最后,我们通过调用th1.start()和th2.start()方法来启动线程。当程序运行时,将会同时输出“hello,world”和“hi”,每隔1秒输出一次,直到输出10次“hello,world”和5次“hi”后,两个线程将会自动退出。
相关推荐
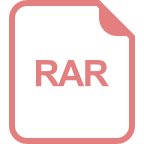
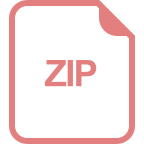
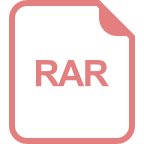





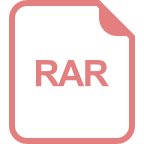
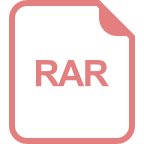
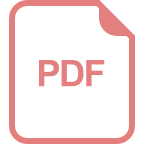
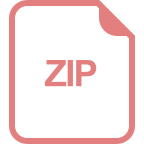
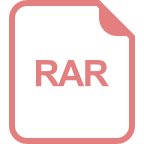
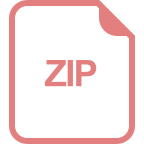